Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial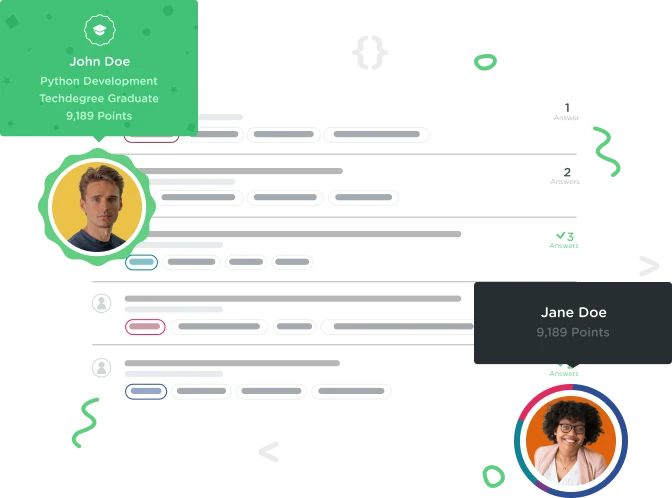
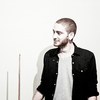
Alex Yakir
5,425 PointsGetting the current location in "Stormy" app
Hey guys, Im trying to implement a current location function in the "Coordinate.swift" of the "Stormy" app.
However I don't want to have to initialize the Coordinate class with "fake" coordinates before I call the getCurrentLocation()
function.
Any Idea how to accomplish this?
The class looks like this:
import Foundation
import CoreLocation
class Coordinate: NSObject, CLLocationManagerDelegate{
var latitude: Double
var longitude : Double
init(latitude: Double, longitude : Double) {
self.latitude = latitude
self.longitude = longitude
}
func getCurrentLocation(){
let locManager = CLLocationManager()
locManager.delegate = self
var currentLocation = CLLocation()
if( CLLocationManager.authorizationStatus() == CLAuthorizationStatus.authorizedWhenInUse ||
CLLocationManager.authorizationStatus() == CLAuthorizationStatus.authorizedAlways){
currentLocation = locManager.location!
}
self.latitude = currentLocation.coordinate.latitude
self.longitude = currentLocation.coordinate.longitude
}
}
extension Coordinate{
override var description: String {
return "\(latitude),\(longitude)"
}
}
I don't want to do this:
let coordinate = Coordinate(latitude: 00.0, longitude: 00.0)
coordinate.getCurrentLocation()
2 Answers

Joe Mayfield
10,409 PointsI've done a tutorial where you create a weather app with location services and I think I had to tell the simulator to use locations that I provided. I went through the simulator and setup some but it gives you apple HQ standard.
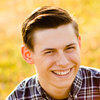
Caleb Kleveter
Treehouse Moderator 37,862 PointsI would create a different model that would handle the fetching of the location that uses delegation to pass on a Coordinate
object that it creates when a new location is detected.
import Foundation
import CoreLocation
protocol LocationManagerDelegate: class {
// Passing in a coordinate to the delegate method
func locationDidUpdate(with location: Coordinate)
}
class LocationManager: NSObject {
let locationManager = CLLocationManager()
weak var delegate: LocationManagerDelegate?
override init() {
super.init()
self.locationManager.delegate = self
self.locationManager.desiredAccuracy = kCLLocationAccuracyThreeKilometers
self.locationManager.requestLocation()
}
func getUserPermission() {
if CLLocationManager.authorizationStatus() == .notDetermined || CLLocationManager.authorizationStatus() == .denied {
locationManager.requestWhenInUseAuthorization()
}
}
}
extension LocationManager: CLLocationManagerDelegate {
func locationManager(_ manager: CLLocationManager, didChangeAuthorization status: CLAuthorizationStatus) {
if status == .authorizedAlways {
manager.requestLocation()
}
}
func locationManager(_ manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
if let location = locations.first {
// Create the coordinate from the location
let coordinate = Coordinate(latitude: location.coordinate.latitude, longitude: location.coordinate.longitude)
// Pass the coordinate into the delegate method
self.delegate?.locationDidUpdate(with: coordinate)
}
}
func locationManager(_ manager: CLLocationManager, didFailWithError error: Error) {
print(error.localizedDescription)
}
}
Conforming to the LocationManagerDelegate
:
class VIEW-CONTROLLER {
let locationManager = LocationManager()
// Other properties...
override viewDidLoad() {
super.viewDidLoad()
locationManager.delegate = self
}
// Other methods...
}
extension VIEW-CONTROLLER: LocationManagerDelegate {
func locationDidUpdate(with location: Coordinate) {
// Use the coordinate you fetched.
}
}