Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial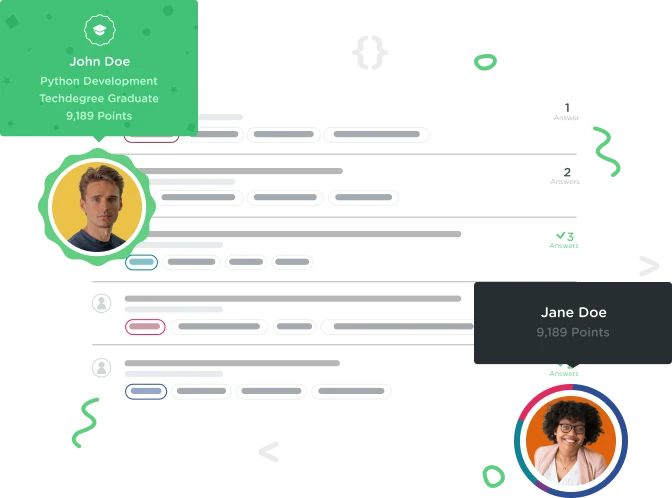

Charlie Prator
Front End Web Development Techdegree Graduate 19,854 PointsGetting the error "SyntaxError: Unexpected end of JSON input"--instead of "Unhandled 'error' event"
To be clear, I followed Andrew Chalkley's video and intentionally put in 'www' into the get request's URL. In the video, Andrew says we're supposed to get the error: Unhandled 'error' event
(see the following video at 1:24: ), but I'm getting the following:
treehouse:~/workspace$ node app.js davemcfarland
undefined:1
SyntaxError: Unexpected end of JSON input
at JSON.parse (<anonymous>)
at IncomingMessage.response.on (/home/treehouse/workspace/app.js:32:28)
at emitNone (events.js:111:20)
at IncomingMessage.emit (events.js:208:7)
at endReadableNT (_stream_readable.js:1064:12)
at _combinedTickCallback (internal/process/next_tick.js:139:11)
at process._tickCallback (internal/process/next_tick.js:181:9)
Below is my JS:
// Problem: We need a simple way to look at a user's badge count and JavaScript points
// Solution: Use Node.js to connect to Treehouse's API to get profile information to print out
// 1. Connect to the API URL (https://teamtreehouse.com/charlieprator.json)
// 1a. Abstract out the username https://teamtreehouse.com/[userName].json)
// 2. Read the data
// 3. Parse the data
// 4. Print the data
// Require HTTPS module
const https = require('https');
// Function: to pring message to console
function printMessage (username, badgeCount, points) {
const message = `${username} has ${badgeCount} total badge(s) and ${points} points in Javascript.`
console.log(message);
}
function getProfile(userName) {
// Connect to the API url $
const request = https.get(`https://wwwteamtreehouse.com/${userName}.json`, response => {
let body = "";
// Read the data
response.on('data', data => {
body += data.toString();
});
// Parse the data
response.on('end', ()=> {
const profile = JSON.parse(body)
printMessage(userName, profile.badges.length, profile.points.JavaScript );
});
response.on('error', error => console.error(`Problem with request: ${error.message}`));
});
}
const users = process.argv.slice(2)
users.forEach(getProfile);
Any idea why?
5 Answers

Carl Orre
5,566 PointsI had the same problem. In order to get the same error message as in the video, just change www to basically anything else. I think that the reason for the error is that in the time since the release of the video they have bought the domain wwwteamtreehouse.com which redirects to the home page. The home page does not have JSON data, resulting in a JSON related error and not the desired Unhandled 'error' event.

Miguel Adan
5,370 Pointscouple more things i've caught.
- your last response.on call is actually suppose to be a request.on call.
- you have it placed lower in the brackets than it should be so move it down two lines past the "});"
- I dont know why this is buy when your going for a bad URL I see you use "www" try using "wwww" instead. I'm not sure how or why it matters but I've found that it does.

Loris Yu
6,294 PointsAfter adding one more "w" to "wwww" I finally get the result same with the video. Thanks!
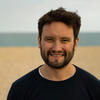
Matt Roberts
5,173 Pointsyes www seems to not work but wwww or other characters does.

Miguel Adan
5,370 PointsI'm watching this like a hawk. I'm having the same issue.
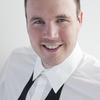
Andrew Reynolds
Front End Web Development Techdegree Graduate 23,333 PointsCharlie Prator,
I had same problem here. I was unable to get the same results as per the video lesson and ended up with the same Syntax error: Unexpected end of JSON input.
The only way I was able to catch the "emitted error" was by using the response.statusCode in an if statement - there is probably a better way but I just testing how to catch emitted errors. If the connection wasn't successful with a 200 code it threw a new error object to be caught.
But it would be great if someone was able to explain why the results couldn't be replicated as per the lesson.
function printMessage(username, badgeCount, points){
const message = `${username} has ${badgeCount} total badge(s) and ${points} points in JavaScript`;
console.log(message);
}
function getProfile(userName){
try{
const https = require('https');
const request = https.get(`teamtreehouse.com/${userName}.json`, response => {
if (response.statusCode === 200){
let body = '';
response.on('data', (d)=> body += d.toString());
response.on('end', ()=>{
const profile = JSON.parse(body);
printMessage(userName, profile.badges.length, profile.points.JavaScript);
});
} else {
throw new Error("incorrect url");
}
});
} catch (error){
console.error(error.message);
}
}
const users = process.argv.slice(2);
getProfile(users);

Miguel Adan
5,370 Pointsidk if its relevant for you but did you notice your missing a semi colon on your
const profile line? around line 33.

Charlie Prator
Front End Web Development Techdegree Graduate 19,854 PointsUpdated it with the semicolon but no dice.
Michal Majewski
Full Stack JavaScript Techdegree Graduate 18,941 PointsMichal Majewski
Full Stack JavaScript Techdegree Graduate 18,941 PointsThanks for this fix!