Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial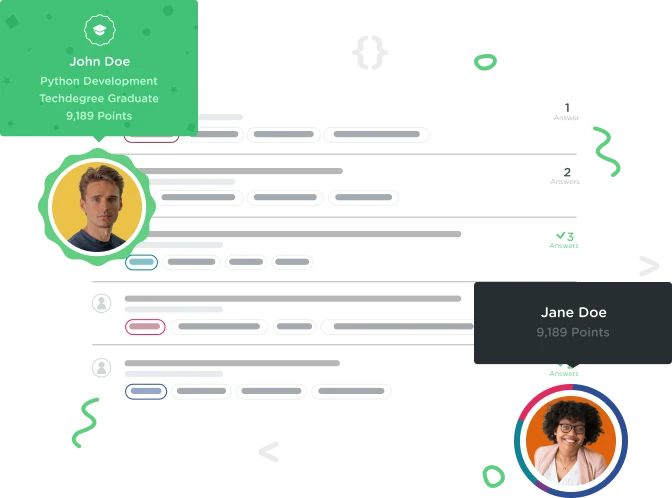

Joanna Suau
3,754 PointsGetting the "img not defined" reference error in the console
Hi, I have followed along and I think I have the exact same code as the teacher, and yet my console logs an error on line 48. The current behavior of the app is as follows:
- it loads a random image
- it loads a list of breeds in the selection box
- the template literal used the text below the image doesn't change and only says affenpinschers
- the user can't select a breed from the selection box
Here's my code:
const select = document.getElementById('breeds');
const card = document.querySelector('.card');
const form = document.querySelector('form');
// ------------------------------------------
// FETCH FUNCTIONS
// ------------------------------------------
function fetchData(url) {
return fetch(url)
.then(res => res.json())
}
fetchData('https://dog.ceo/api/breeds/list')
.then(data => generateOptions(data.message))
fetchData('https://dog.ceo/api/breeds/image/random')
.then(data => generateImage(data.message))
// ------------------------------------------
// HELPER FUNCTIONS
// ------------------------------------------
function generateOptions(data) {
const options = data.map(item => `
<option value='${item}'>${item}</option>
`).join('');
select.innerHTML = options;
}
function generateImage(data) {
const html = `
<img src='${data}' alt>
<p>Click to view images of ${select.value}s</p>
`;
card.innerHTML = html;
}
function fetchBreedImage() {
const breed = select.value;
const image = card.querySelector('img');
const p = card.querySelector('p');
fetchData(`https://dog.ceo/api/breed/${breed}/images/random`)
.then(data => {
img.src = data.message;
img.alt = breed;
p.textContent = `Click to view more ${breed}s`;
})
}
// ------------------------------------------
// EVENT LISTENERS
// ------------------------------------------
select.addEventListener('click', fetchBreedImage);
card.addEventListener('click', fetchBreedImage);
// ------------------------------------------
// POST DATA
// ------------------------------------------
2 Answers
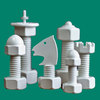
Steven Parker
241,807 PointsIn "fetchBreedImage", a variable named "image" is defined; but references are made to "img", which is not defined.
And to make it easier to replicate your issue in future questions, make a snapshot of your workspace and post the link to it here.

Joanna Suau
3,754 PointsNow this is embarrassing :) thank you so much for your help!
Joanna Suau
3,754 PointsJoanna Suau
3,754 PointsHi Steven,
thank you for your reply and a link to snapshot taking explanation. Will definitely come in handy!
Regarding my original issue, I thought that this query selector targets any img html tag used in the card div just like the one below for the p tag? the console doesnt log any errors for the p tag. Could you help me a bit more please?
Steven Parker
241,807 PointsSteven Parker
241,807 PointsThe issue is the name of the variable that the query selector is assigned to: