Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial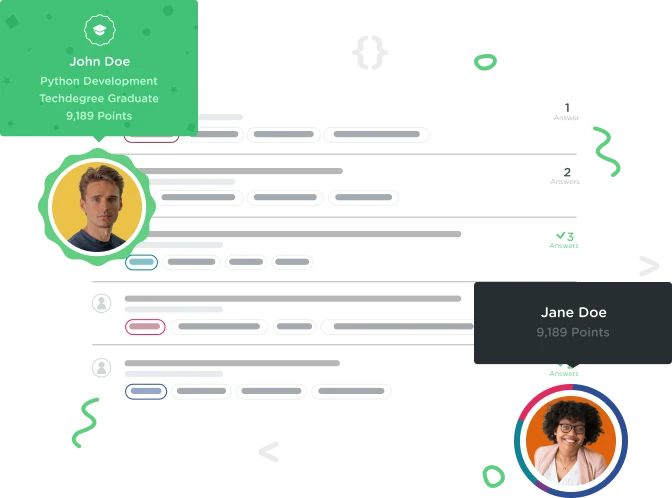

Lerena Holloway
9,855 PointsGetting the number of values in dictionary challenge
I'm printing the key that has the highest value. I've ran and tested the code in my terminal, but for some reason, it won't work in the treehouse portal. I'm not sure where I'm going wrong. It doesn't give any indications other than saying that the right key is not being returned.
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(dict1):
num_teachers = 0
for key in dict1.keys():
num_teachers += 1
return num_teachers
def num_courses(dict1):
num_values = 0
for values in dict1.values():
for item in values:
num_values += 1
return num_values
def courses(dict1):
num_courses = []
for values in dict1.values():
for item in values:
num_courses.append(item)
return num_courses
def most_courses(dict1):
value1 = []
for key, value in dict1.items():
value1.append(len(value))
if len(value) == max(value1):
return key
1 Answer
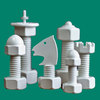
Steven Parker
229,732 PointsSince you build "value1" one element at a time, you probably won't want to return based on a test inside the loop. Instead, you could just identify the largest in the loop and return the associated name after the loop finished.
Lerena Holloway
9,855 PointsLerena Holloway
9,855 PointsThank you! That did the trick. I had to remove the "if" statement from the loop.