Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial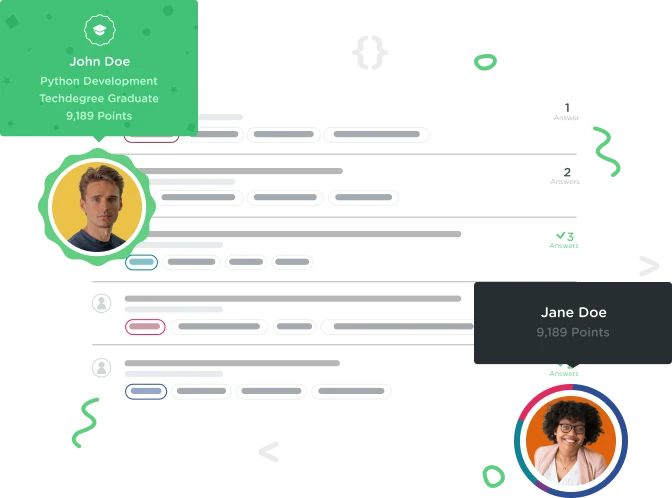

Lauren Haygood
16,901 PointsGetting the wrong error message on POST.
Problem:
When I post the user sign up form, it always triggers the first else
statement, which means it doesn't think all the required fields are there, even when they are. I confirmed that they are being submitted in the Chrome dev tools by inspecting the network call and sure enough, all the fields are being submitted.
Code:
// register form
form(method='POST' action='/api/register')
div.form-group
label(for='name') Name:
input#name.form-control(type='text', placeholder='first and last' name='name')
div.form-group
label(for='email') Email:
input#email.form-control(type='email', placeholder='name@email.com' name='email')
div.form-group
label(for='favoriteBook') Favorite Book:
input#favoriteBook.form-control(type='text', placeholder='title of book' name='favoriteBook')
div.form-group
label(for='pw') Password:
input#pw.form-control(type='password' name='password')
div.form-group
label(for='pw2') Confirm Password:
input#pw2.form-control(type='password' name='confirmPassword')
button.btn.btn-primary(type='submit') Sign up
// POST /register
router.post('/register', function(req, res, next) {
if (req.body.email &&
req.body.name &&
req.body.favoriteBook &&
req.body.password &&
req.body.confirmPassword) {
// confirm that user typed same password twice
if (req.body.password !== req.body.confirmPassword) {
var err = new Error('Passwords do not match.');
err.status = 400;
return next(err);
}
// create object with form input
var userData = {
email: req.body.email,
name: req.body.name,
favoriteBook: req.body.favoriteBook,
password: req.body.password
};
// use schema's `create` method to insert document into Mongo
User.create(userData, function (error, user) {
if (error) {
return next(error);
} else {
return res.redirect('/profile');
}
});
} else {
var err = new Error('All fields required.');
err.status = 400;
return next(err);
}
});
So, this route has two nested IF
blocks, minus the code for adding it to Mongodb. For some reason it always gets caught in the first else
block no matter what I submit. Any ideas why?
2 Answers

Lauren Haygood
16,901 PointsThe issue was in my server.js
file. The req.body
was always blank because it didn't have these lines in the file:
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({ extended: false }));
So, it wasn't parsing the form data properly.

Thomas Nilsen
14,957 Pointsconsole log out everything as the first thing you do in the POST request. Some of them is probably null or undefined due to some minor misspell I'm guessing.
console.log([req.body.email,
req.body.name,
req.body.favoriteBook,
req.body.password,
req.body.confirmPassword]);
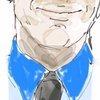
Abraham Juliot
47,353 PointsGood tip. Thank you!
Thomas Nilsen
14,957 PointsThomas Nilsen
14,957 PointsAh - Easy to forget.