Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial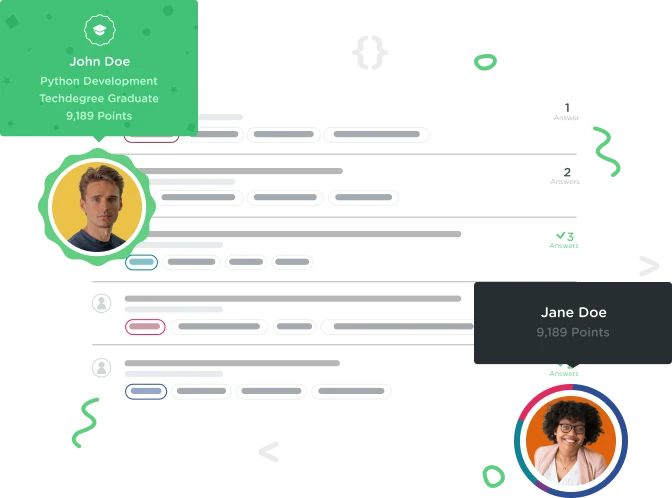

Brad Pettigrew
1,834 PointsGetting total minutes as a timedelta
I'm stuck on this exercise. I think the problem is converting the total minutes in each datetime to a timedelta, subtracting that number, and then converting it back to minutes.
How would I do this?
import datetime
def minutes(dt1,dt2):
diff = dt2.minute - dt1.minute
return round(diff)
2 Answers
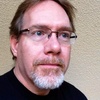
Chris Freeman
Treehouse Moderator 68,460 PointsIn your code, you are subtracting the integer minute values to get another integer. This does not take into account if the datetimes cross the hour boundary. It would be better to subtract the two datetime objects directly to yield a timedelta object. You can then use the timedelta method total_seconds()
and covert back to minutes.

Jonathan Shull
Courses Plus Student 12,739 PointsI struggled with this one some as well. Using total_seconds(), as Chris Freeman states above, was the key for me.
A little more detail into how I solved the problem, each step adds on to the previous:
First I subtracted the the function's arguments to create a timedelta:
date_time_newer - date_time_older
Next I converted the timedelta (in the form 06:45) to seconds using total_seconds():
(date_time_newer-date_time_older).total_seconds()
Then I divided everything by 60 and rounded it to get total minutes:
round((date_time_newer-date_time_older).total_seconds()/60)
In the end I ended up with:
import datetime
def minutes(date_time_older, date_time_newer):
date_time_difference = round((date_time_newer-date_time_older).total_seconds()/60)
return date_time_difference
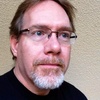
Chris Freeman
Treehouse Moderator 68,460 PointsJonathan, your original answer with the details was fine. I was going to upvote for the details you added. Feel free to edit your answer and add that back in if you still have it.