Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial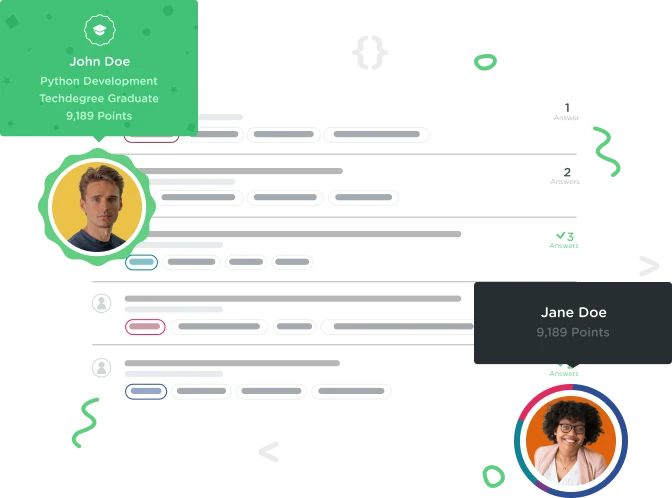

Aaron Hill
9,732 PointsGetting TypeError when trying to create status code errors.
I'm on the part where we add to the "if" statement in the event of a status code error. This is my code:
//Problem: We need a simple way to look at user's badge count and javascript points.
//Solution: Use Node.JS to connect to treehouses api.
var https = require("https");
var username = "aaronhill34";
//Print out message
function printError(error){
console.error(error.message);
}
function printMessage(username, badgecount, points) {
var message = username + " has " + badgecount + " total badge(s) and " + points + " points "
;
console.log(message);
}
//Print out error messages
//Connect to API Url
var request = https.get("https://teamtreehouse.com/" + username + ".json", function(response){
var body = "";
//Read the data from the response
response.on('data', function(chunk) {
body += chunk;
});
response.on("end", function(){
if(response.statusCode === 200) {
try {
var profile = JSON.parse(body);
printMessage(username, profile.badges.length, profile.points.JavaScript)
} catch(error) {
//Parse Error
printError(error);
}
} else {
//Status Code Error
printError({message: "There was an error getting the profile for " + username + ". (" + https.STATUS_CODES[response.statusCode] + ")"});
}
});
//parse data
//print the data out
});
//Connection Error
request.on("error", printError);
It throws an error in the console but instead of a nice string I get the following:
treehouse:~/workspace$ node app.js
/home/treehouse/workspace/app.js:38
or getting the profile for " + username + ". (" + https.STA
TUS_CODES[response.
TypeError: Cannot read property '404' of undefined
at IncomingMessage.<anonymous> (/home/treehouse/workspa
ce/app.js:38:112)
at IncomingMessage.emit (events.js:117:20)
at _stream_readable.js:944:16
at process._tickCallback (node.js:442:13)
Is it because I'm using https instead of http? I thought I had to use https because when I use http I get a different error. I appreciate any help you can offer.
5 Answers

Aaron Hill
9,732 PointsI found a workaround,
I guess you can't use .STATUS_CODES when accessing the https object. So that's why I was getting the typeError. To fix it I changed the object to http.STATUS_CODES and made sure to require http in addition to https at the top of my code.
"Great job, me!" "Thanks, me!"

Jeff Lau
13,627 Pointsyeah there is no https.STATUS_CODES object thats why you are getting a type error of undefined (basically telling you that https.STATUS_CODES doesn't exist).
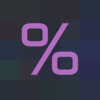
R Halabi
587 PointsThanks, guys! This was the perfect fix.
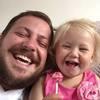
Jamie Tuffen
8,042 PointsYeah worked well for me. Thanks.

Henri CLOCUH
31,209 PointsThanks! It works.