Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial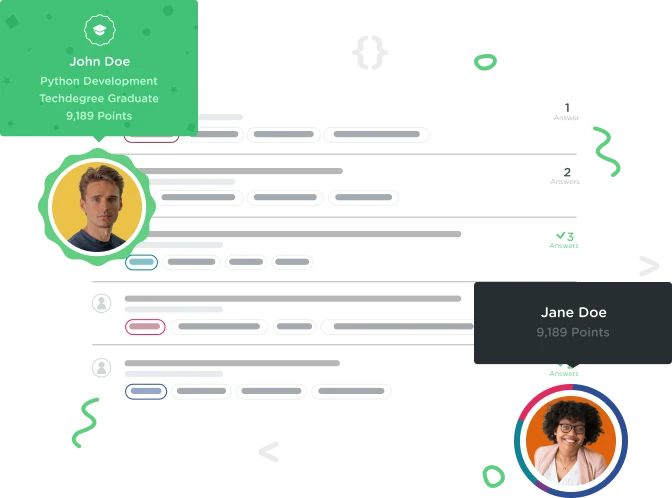
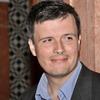
Brian Patterson
19,588 PointsGetting Uncaught ReferenceError: QuizUI is not defined at app.js:11
I can figures out why I am getting this error
Uncaught ReferenceError: QuizUI is not defined
at app.js:11
Below is my code for the UI
var QuizUI = {
displayNext: function () {
if (quiz.hasEnded()) {
this.displayScore();
} else {
this.displayQuestion();
this.displayChoices();
this.displayProgress();
}
},
displayQuestion: function() {
this.populateIdWithHTML("question", quiz.getCurrentQuestion().text);
},
displayChoices: function() {
var choices = quiz.getCurrentQuestion().choices;
for(var i = 0; i < choices.length; i++) {
this.populateIdWithHTML("choice" + i, choices[i]);
this.guessHandler("guess" + i, choices[i]);
}
},
displayScore:function() {
var gameOverHTML = "<h1>Game Over</hi>";
gameOverHTML += "<h2> Your score is: " + quiz.score + "</h2>";
this.populateIdWithHTML("quiz", gameOverHTML);
},
populateIdWithHTML: function(id, text) {
var button = document.getElementById(id);
button.onclick = function() {
quiz.quess(guess);
QuizUI.displayNext();
}
},
guessHandler: function(id, guess) {
var button = document.getElementById(id);
button.onclick = function() {
quiz.guess(guess);
QuizUI.displayNext();
}
}
displayProgress: function() {
var currentQuestionNumber = quiz.currentQuestionIndex + 1;
this.populateIdWithHTML("progress", "Question " + currentQuestionNumber + " of " + quiz.questions.length);
}
};
Plus this is my code for the app.js
//Create Questions
var questions = [
new Question("Who was the first President of the United States?", [ "George Washington", "Thomas Jefferson" ], "George Washington"),
new Question("What is the answer to the Ultimate Question of Life, the Universe, and Everything?", ["Pi","42"], "42")
];
//Create Quiz
var quiz = new Quiz(questions);
//Display Quiz
QuizUI.displayNext();
Plus, I am getting an uncaught syntax error on line 41 for the ui. Any help would be appreciated.
3 Answers
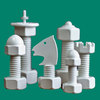
Steven Parker
231,275 PointsWithout testing it in the actual context, I would expect you might need to explicitly pass "this" and both the item and the index to get the same behavior:
choices.forEach(function(choice, i) {
this.populateIdWithHTML("choice" + i, choice);
this.guessHandler("guess" + i, choice);
}, this);
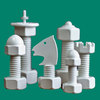
Steven Parker
231,275 PointsThe issue is likely to be in the HTML file. The code for "app.js" is being loaded, but it looks like "QuizUI.js" is not.
If you have trouble pinning down the issue, make a snapshot of your workspace and post the link to it here.
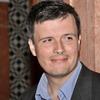
Brian Patterson
19,588 PointsI figured it out, Steven. I had legacy code that was interfering with the code he had. I do have one other question though. I am trying to refactor the for-loop. From this:
for(var i = 0; i < choices.length; i++) {
this.populateIdWithHTML("choice" + i, choices[i]);
this.guessHandler("guess" + i, choices[i]);
}
to this:
choices.forEach(function(choice){
this.populateIdWithHTML("choice" + choice, choice);
this.guessHandler("guess" + choice, choice)
});
What is the best what to get this to work with the latest forEach helper for JavaScript? Thanks for your help.
Brian Patterson
19,588 PointsBrian Patterson
19,588 PointsThanks for that Steven. Two questions why
this
after the function? Also, I see you have been really helpful on the forums what do you think is the best way to learn? Its one thing understanding it but a completely different ball game apply it.Steven Parker
231,275 PointsSteven Parker
231,275 PointsI think it mostly comes down to practice. The more you use the information you learn, the longer you will retain it and the better you will become at knowing when and how to apply it.