Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial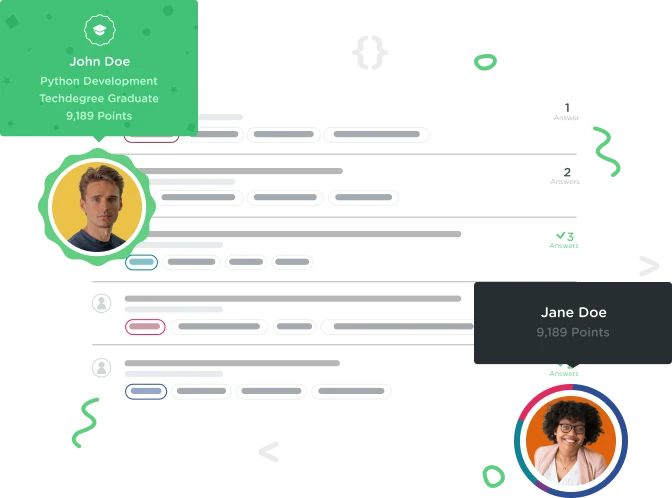
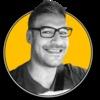
Fabio Picciau
5,923 PointsGetting Uncaught TypeError when adding {this.onSubmit} (React Basics)
I added the code to the AddPlayerForm class
const AddPlayerForm = React.createClass({
onSubmit: (e) => {
e.preventDefault();
},
render: () => {
return (
<div className="add-player-form">
<form onSubmit = {this.onSubmit}>
<input type="text" />
<input type="submit" value="Add Player" />
</form>
</div>
);
}
the problem is that I keep getting an Uncaught TypeError for {this.onSubmit}
app.jsx:28 Uncaught TypeError: Cannot read property 'onSubmit' of undefined
at Constructor.render (eval at transform.run (babel-browser.min.js:4), <anonymous>:31:30)
at ReactCompositeComponentWrapper._renderValidatedComponentWithoutOwnerOrContext (react.js:6157)
at ReactCompositeComponentWrapper._renderValidatedComponent (react.js:6177)
...
I cannot find the bug unfortunately
Help is much appreciated thanks
3 Answers

Sarah Saad
Courses Plus Student 3,223 PointsHi,
Problem is that you are using arrow function syntax for method functions which is not correct.
Arrow functions are recommended to be using only for non-method functions. I have updated your code as below and it's working.
import React from 'react';
const AddPlayerForm = React.createClass({
onSubmitAutoBinded: function(e){ e.preventDefault; console.log("form submitted"); },
render: function() { return ( <div className="add-player-form"> <form onSubmit = {this.onSubmitAutoBinded}> <input type="text" /> <input type="submit" value="Add Player" /> </form> </div> ); }
});
export default AddPlayerForm;

Sarah Saad
Courses Plus Student 3,223 PointsActually you only need to keep the render() method as below. OnSubmit function is fine as a arrow function.
onSubmit: (e) => {
e.preventDefault();
},
render: function() {
return (
<div className="add-player-form">
<form onSubmit = {this.onSubmit} id="addPlayerForm">
<input type="text" />
<input type="submit" value="Add Player" />
</form>
</div>
);
}
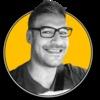
Fabio Picciau
5,923 PointsThank you very much Sarah! I forgot about the non-method function "rule"