Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial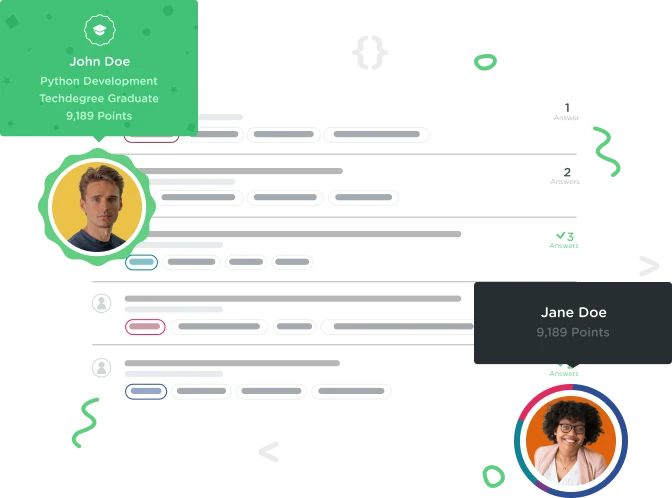

Haziq Mir
Courses Plus Student 4,656 PointsGetting "undefined" points when trying to access points for a topic.
I am trying to perfect the app as suggested in the teacher's notes. I can't, however, get the app to fetch points in a topic specified by the user. Here is my code:
// index.js
var profile = require("./profile");
var topic = process.argv.slice(2,3);
var users = process.argv.slice(3);
if (users.length > 0) {
users.forEach(function(user){
profile.get(user, topic);
});
} else {
console.log("Please type in a user name, like this: node index.js <username1>, ...");
}
// index.js
var profile = require("./profile");
var topic = process.argv.slice(2,3);
var users = process.argv.slice(3);
if (users.length > 0) {
users.forEach(function(user){
profile.get(user, topic);
});
} else {
console.log("Please type in a user name, like this: node index.js <username1>, ...");
}
// profile,js
var http = require("http");
// Print out message
function printMessage(username, badgeCount, points, topic) {
var message = username + " has " + badgeCount + " total badge(s) and " + points + " points in " + topic + ".";
console.log(message);
}
// Print out error
function printError(error) {
console.error(error.message);
}
function getProfile(username, topic) {
var request = http.get("http://teamtreehouse.com/" + username + ".json", function(response){
// console.log(response.statusCode);
var body = "";
response.on('data', function(chunk) {
body += chunk;
});
response.on('end', function() {
if (response.statusCode === 200) {
try {
// Parse the data
var profile = JSON.parse(body);
// Print the data
printMessage(profile.name, profile.badges.length, profile.points.topic, topic);
} catch(error) {
// Parse Error
printError(error);
}
} else {
// Status Code error
printError({message: "There was an error getting the profile for " + username + ". (" + http.STATUS_CODES[response.statusCode] + ")"});
}
});
});
// Connection Error
request.on('error', printError);
}
module.exports.get = getProfile;
1 Answer
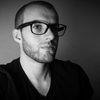
Stefan Osorio
16,419 PointsHi Haziq!
The problem lies in this line:
printMessage(profile.name, profile.badges.length, profile.points.topic, topic);
You are using dot notation to access the points of the selected topic. Dot notation is great because it's fast to write and easy to read, but in certain cases you have to use square bracket notation. Selection of properties with variables ("topic") is one of those cases.
This should work:
printMessage(profile.name, profile.badges.length, profile.points[topic], topic);
Andrew Chalkley
Treehouse Guest TeacherAndrew Chalkley
Treehouse Guest TeacherThe square bracket notation is helpful when you want to programmatically access the key of an object for example...
If you use dot notation
person.key
isundefined
because it's looking for something like this:So the square brackets are specifically used for these type of dynamically accessed values. Dot notation is used when you know the key you want to access and it's not dynamic (for example from user input).
Haziq Mir
Courses Plus Student 4,656 PointsHaziq Mir
Courses Plus Student 4,656 PointsThanks for the help, guys! :)