Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial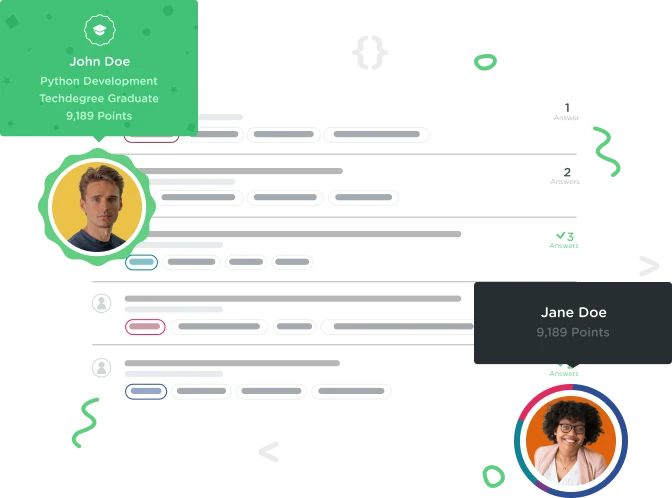

ammarkhan
Front End Web Development Techdegree Student 21,661 Pointsgetting value for the key:value pair.
I am unable to understand and hence pass how to pass value into the loop, from my understand
for(key in value){
console.log(key,':', person[value]);
}
i might be wrong as it is confusing and i cannot understand on how to access key value pair.
var shanghai = {
population: 14.35e6,
longitude: '31.2000 N',
latitude: '121.5000 E',
country: 'CHN'
};
for(var key in shanghai){
console.log(key[shanghai], ':' ,shanghai['key'])
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Objects</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers

Eduardo Parra San Jose
12,579 PointsHi,
I'll try to help. When you use for in to loop through an object, the variable holds the value of the property. So when you do:
var shanghai = {
population: 14.35e6,
longitude: '31.2000 N',
latitude: '121.5000 E',
country: 'CHN'
};
for(var key in shanghai){
}
In the first iteration key will be "population", in the next iteration key will be "longitude" and so on. In order to log the property and value to the console:
// as key hold the value of the property
// shangai[key] will give you the value for that property
for(var key in shanghai){
console.log(key, ":", shanghai[key]);
}
The final code will look like:
var shanghai = {
population: 14.35e6,
longitude: '31.2000 N',
latitude: '121.5000 E',
country: 'CHN'
};
for(var property in shanghai) {
console.log(property, ":", shanghai[property]);
}
I hope it helps. Have a nice day :)
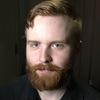
Nicholas Vogel
12,318 PointsThe solution is pretty simple, and it follows the formula you provided in your first code block. Instead of putting
console.log(key[shanghai]))
it should just be
console.log(key, ':', shanghai[key]);
Note: you also forgot a ; at the end of your console.log and the key in the square bracket shouldn't have quotes. It looks like you understand this from your first code block, but feel free to ask more questions!
ammarkhan
Front End Web Development Techdegree Student 21,661 Pointsammarkhan
Front End Web Development Techdegree Student 21,661 PointsWhy can't we use + to concat javascript variable, why use , ":",?