Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial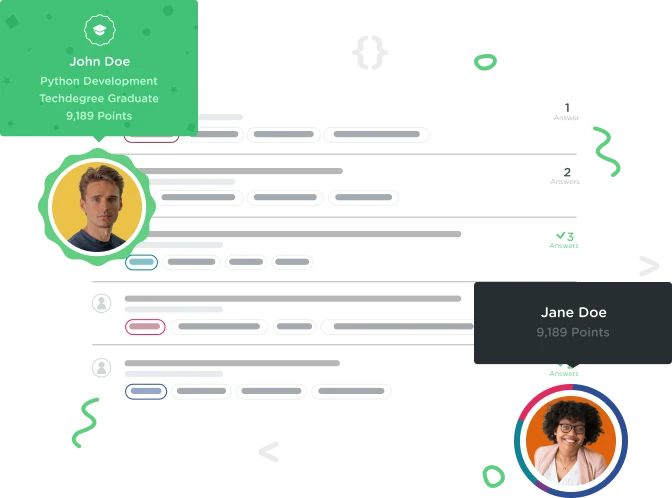

leonard jurado
12,445 Points.get(word,0)+1
Hi guys,
I've just passed the word_count test, not because I was able to think my way out of it, but because I saw in some Q&A here the last bit of code that uses this script .get(word,0)+1
Basically, the whole code is:
def word_count(string1):
string2 = string1.lower()
list1 = string2.split()
dict1 = {}
for word in list1:
dict1[word]=dict1.get(word,0)+1
return(dict1)
Problem is, I've already memorized the code without really understanding the portion which says:
dict1[word]=dict1.get(word,0)+1
Can someone explain it to me please?
Thanks
5 Answers
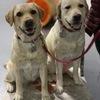
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Leonard,
To understand how the dict1.get(word,0)
is working, let's first consider the alternative.
We know that any time we encounter a particular word in the list, we want to look up that word in the dictionary, and increment the value by 1.
We can look up a word in a dictionary as follows:
dict1[word]
Which is all well and good so long as word is already in the dictionary. If it isn't, we get a crash. We can avoid the crash by using an if
statement to check if word is in dict1 and create that key if it doesn't already exist.
Alternatively we can use the get()
method on a dictionary. In its simplest form we do the following:
dict.get(word)
If word is in the dictionary, we get the same result as if we had accessed it using subscripting. But if the word isn't already in the dictionary, we don't get a crash, we get None.
That's better, because we're not crashing outright, but it doesn't help us with the incrementing the value part of the solution:
dict1[word] = dict.get(word) + 1
In the above case, everything works as we expect when we increment a key that already has a value. For example, let's say that our dictionary looks like this:
list = {'hello': 1, 'world': 1}
When word is 'hello', the code above will get
the value for the key 'hello', which is 1
and add 1 to it, then put that value back into the dictionary at the key 'hello' (so list will now be {'hello': 2, 'world': 1}.
The problem arises when we get to the first occurrence of a new word, let's say the word is 'python', dict.get(word)
will look for 'python' in the dictionary, not find it, and evaluate to None. Because you can't add an int to None, we'll get a crash (TypeError).
This is where the second argument to get()
comes in. As the documentation reveals:
get(key[, default]) Return the value for key if key is in the dictionary, else default. If default is not given, it defaults to None, so that this method never raises a KeyError.
We can supply a default value for when the key is not found in the dictionary (instead of None). This means that by coding the following:
dict1[word] = dict.get(word, 0) + 1
The first time word
is 'python' we now evaluate the dict.get()
to 0 (instead of None). We can add 1 to 0 (to get 1) and so we are able to insert that key into the dictionary with the correct starting value.
Hope that clears everything up for you.
Cheers
Alex

weiquanlo
12,054 PointsThere are another simply answer by using "list.count()"
def word_count1(string1):
string2 = string1.lower()
list1 = string2.split()
dict1 = {}
for word in list1:
dict1[word] = list1.count(word)
return dict1
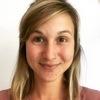
Chels .
4,720 PointsWei Quan Lo Thanks for sharing!! I think .count() makes more sense for where we are in the course
** except it doesn't work for the code challenge, not sure why?
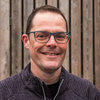
Chris Guy
Python Web Development Techdegree Graduate 14,615 PointsThanks for this suggestion Wei Quan Lo - it makes sense to me and passes .the code challenge.
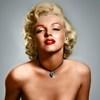
Shane Finlayson
13,174 PointsThank you Alex, you may have saved a life today! I was about to loose it on this one!
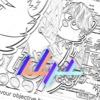
camberden
2,729 PointsI second that thanks! I've been grounded in my python course for quite a while due to this problem.

leonard jurado
12,445 PointsHi Alex,
I was able to understand it thanks to your extensive answer.
Regards,
Leonard

Firuz Abduganiev
3,140 Pointsdef word_count(sentence):
dict = {}
sentence = sentence.split()
for word in sentence:
x = sentence.count(word)
dict.update({word.lower(): x})
return dict
Oskar Lundberg
9,534 PointsOskar Lundberg
9,534 PointsReally appreciated this answer :D