Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial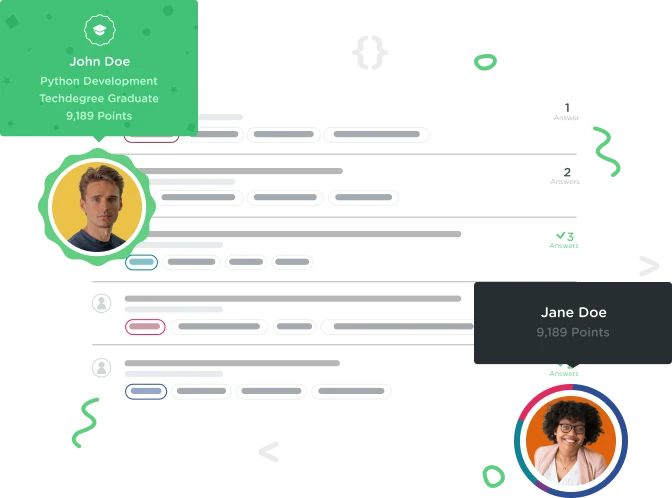

Rico Petrini
431 Pointsgive me the answer please ive spent hours on this
thx forur time
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter = 0 {
print("k")
counter <= 0
}
1 Answer
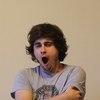
Arman Arutyunov
21,900 PointsHello!
In this task you are asked to firstly create such a while loop that would iterate until the counter variable is less than amount of items in the numbers array.
To do so you have to compare a counter variable and numbers.count ( .count gives you the amount of items in any array). If counter is less than numbers.count you proceed, if not the block will be over. But to do it right we have to increase the counter by one in each iteration.
You do so like this:
counter = counter + 1
or like this
counter += 1
or even like this
counter++
but this last example is an old way from language C which is not currently working in Swift 3. So let's use this one
counter += 1
which is the cleanest one from the two we've got left.
Then you have to get the value of each element inside the array by an index and add it to the sum variable. To do so let's use our counter variable as an index which will represent each index of the array. It's a good idea because this counter variable will increase from 0 to the last number which is equal to the last index of the array because we've already set our while loop in such a way that it will stop iterating when the counter is greater than the last index of array.
To get a value from numbers array by index which is represented by counter variable you just need to do it like this
let value = numbers[counter]
And to add the value to the sum variable you do it just the way we've increased our counter variable earlier
sum += value
But actually it is not required to store our value inside the new variable so you can do it in one line of code like this
sum += numbers[counter]
and this will be enough.
Hope I didn't create new questions for you rather than answering them)
This is how your challenge result could look like to be passed:
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter < numbers.count {
sum += numbers[counter]
counter += 1
}
Hope it helps. And of course if you have more questions you are free to ask.
Happy coding!