Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial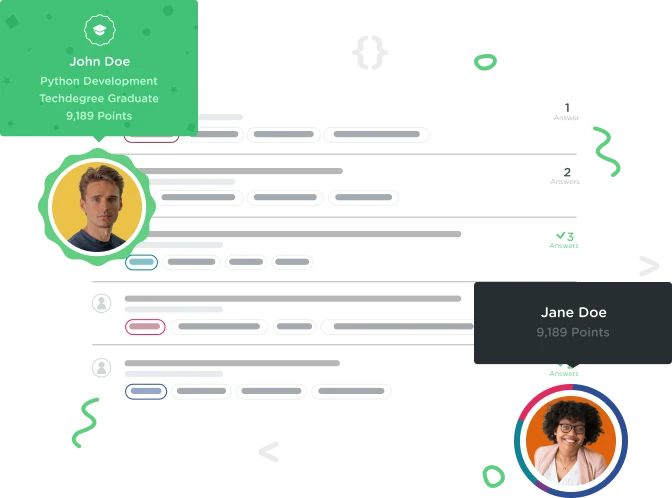

Sejong Consortium
1,406 PointsGiven correct answer and evaluated as wrong.
Challenge Question comments:
E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
{'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
Lowercase the string to make it easier.
def word_count(string):
word_list = string.lower().split(" ")
dict_count = {}
for key in word_list:
if key not in dict_count:
dict_count[key] = word_list.count(key)
return dict_count
My result in iPython is:
"result {'am': 1, 'do': 1, 'i': 2, 'it': 1, 'like': 1, 'not': 1, 'sam': 1}"
and it is considered equivalent to the answer in the comments at the top of the snippet above.
"result == {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1} True"
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(string):
word_list = string.lower().split(" ")
dict_count = {}
for key in word_list:
if key not in dict_count:
dict_count[key] = word_list.count(key)
return dict_count
2 Answers
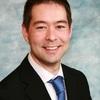
Mark Chesney
11,747 PointsHi Sejong,
I ran this, and I don't know why yours doesn't pass, even though I think that your answer should:
>>> treehouse_answer = {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
>>> sejong_answer = word_count("I do not like it Sam I Am")
>>> treehouse_answer == sejong_answer
True
I've tried my solution, and it seems to work, below. Note that the first loop must be completed **before* the second loop even begins*, even though they iterate over the same list.
def word_count(string):
dict1 = {}
string = string.lower()
list_of_words = string.split()
for word in list_of_words:
dict1[word] = 0
for word in list_of_words:
dict1[word] += 1
return dict1
Your returned dictionary matches mine, so again, I'm sorry I don't know why yours doesn't work, but I hope my code might clear up any confusion.
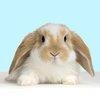
Zara Thomas
7,522 PointsI think you need to add an else statement. That way if there is a repeat word in the string, it will be counted again.

Sejong Consortium
1,406 PointsI don't think so.
The condition 'if key not in dict_count' is meant to check and see if the key (the current word we are counting in the string) exists in the dictionary I am building.
This means, for example, when counting the number of occurences of the word "I", it checks the whole string in one sweep, and adds "I" as a key in the dictionary.
Since dictionary keys must be unique, and since I check before each count to see if a key (previously counted word) already exists in my dictionary, there should be no risk of recounting the word.
Since there is no "else", it should just continue the for loop and skip that word if it is already in the dictionary.
Thank you for your suggestion and feedback! :)
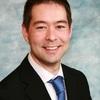
Mark Chesney
11,747 PointsI agree with your response, Sejong. By looking at your code, and running it in my Python environment, it is clear to me that you have a 100% full grasp of the concepts taught in this lesson. I know I've solved this challenge numerous times, each time with different results. I love Treehouse's code challenges but once in a while my code won't work and it's unclear why. Your code demonstrates to me that you know how to use Python and want to learn more -- I hope you keep moving forward with it! :)
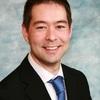
Mark Chesney
11,747 Pointsah, I see: str.split()
will pass the challenge; str.split(" ")
will not pass. Great to know -- thanks Sejong!!
Sejong Consortium
1,406 PointsSejong Consortium
1,406 PointsI read Googled this problem last night and found a solution similar to yours.
You Split on ALL whitespace ( …. .split() ), and I split on regular space ( .split(" ") )
As soon as I changed that, my answer was accepted.
Thanks for taking the time to investigate this with me!
All the best