Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial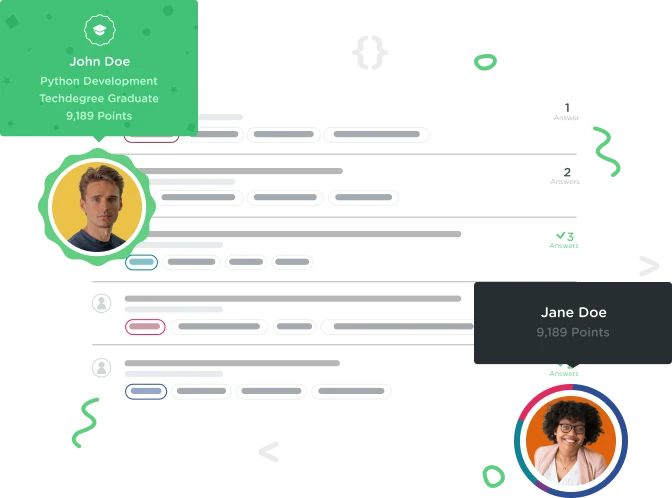

Duke Branding
3,606 PointsGiven that If less than 10 is also less than 100, am i correct that the 2nd Else If condition is higher than the 1st?
We have three If Statements (2 of them being Else If).
The first line asks If < 10, but The second line asks Else If < 100, while The third line asks Else If > 100
Why doesn't the second line supercede, or replace the first line? It seems to only add to the conditions being tested.
Why couldn't we ask
if ( <= 10 )
if ( ( >10) && (<=100) )
How would you explain this in better terms?
Thanks.
2 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Duke,
Here's the code that I think you're asking about:
var caffeine_mg = 62;
var mood;
if (caffeine_mg < 10) {
mood = 'grumpy';
} else if (caffeine_mg < 100) {
mood = 'operational';
} else if (caffeine_mg >= 100) {
mood = 'winning!';
}
When you have an if/ else if structure they're all linked together and only 1 of those will be executed. As soon as the first true condition is met, the code in that block will be executed and the rest of the conditions will be skipped. So if the caffeine level had been 5 for example, then the 1st if
condition would be true and the 2nd else if
condition wouldn't even be checked it would be skipped.
You could make the second else if
condition like you suggested: else if (caffeine_mg >= 10 && caffeine_mg < 100) {
but the first part is unnecessary because you know that if you've made it that far then it has to be greater than or equal to 10.
If you did separate if
blocks then you would need the extra check because separate if
blocks would all be evaluated separately.
var caffeine_mg = 62;
var mood;
if (caffeine_mg < 10) {
mood = 'grumpy';
}
if (caffeine_mg >= 10 && caffeine_mg < 100) {
mood = 'operational';
}
if (caffeine_mg >= 100) {
mood = 'winning!';
}
Even if the first one is true, the 2nd and 3rd will still be evaluated and so you need extra checks in place to guard against more than one of these being true at the same time.

Michael Miller
5,747 PointsThe first line asks if it is less than or equal to 10,
If that evaluates as false then it checks to see if it is less than or equal to 100.
If the first line evaluates true then it will never get to the second or third line.
The third line checks to see if it is greater than 100, although you could just assume that if the first two fail.
Three conditions are being tested for: x = anything less than 10 x = 11 through 100 x = anything greater than 100
if (x <= 10) {
// run if x < 10
} else if (x <= 100) {
// run if x > 10 and x <=100
} else {
// run if x > 100
}
Hope this helps
Duke Branding
3,606 PointsDuke Branding
3,606 PointsI understand it now, thank for your answer. You're right, that was the code I was talking about.
At first I didn't get the nuance between the first two evaluations. Once the first evaluation is seen as false, then the second is "stacked" as an additional evaluation, building the first into the second.
I still don't quite know how to articulate it, but I do understand it now.