Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial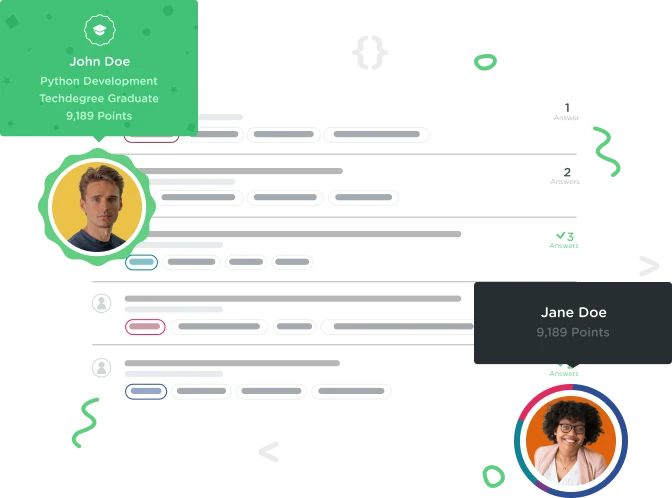
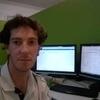
Gavin Mace
30,747 PointsGo Lang -> If Statements -> Code Challenge (Blackjack)
Hey guys,
I'm a little bit confused with this one and I don't think that the course has shown the syntax for how to solve it. I think it's likely that I have errors because I'm using 'fmt.Println()' rather than 'return', but having my error message point me in the direction of syntax error is confusing.
1) Define what "Score" is. Should I do this somewhere? so the function can actually run and deliver a result?
2) Why is my 'else if' statement on line 9 causing a syntax error? All looks fine to me :/
Any help greatly appreciated, thanks!
TASK: Update the PlayHand function to return a string that represents a play appropriate for the current score. If score is less than 17, return "hit me". If score is greater than 21, return "bust". In all other cases, return "stand".
Bummer! # blackjack src/blackjack/player.go:9: syntax error: unexpected else, expecting } FAIL blackjack [build failed]
package blackjack
func PlayHand(score int) string {
if score < 17 {
fmt.Println("hit me")
}
else if score > 21 {
fmt.Println("bust")
}
else {
fmt.Println("stand")
}
}
6 Answers
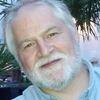
Jeff Muday
Treehouse Moderator 28,720 PointsYou're so close! You got the syntax right, but Go is a little more picky about some of its flow-control token and brace placement.
Go's "if" statement is dependent on keeping the braces on the same line as its preceding token (I reformatted your code below). Also, I don't recall if this challenge is supposed to have a return value, so I removed that from the "func PlayHand" declaration.
Best of luck with Golang!
func PlayHand(score int) {
if score < 17 {
fmt.Println("hit me")
} else if score > 21 {
fmt.Println("bust")
} else {
fmt.Println("stand")
}
}
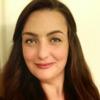
Jennifer Nordell
Treehouse TeacherHi there! Jeff Muday is absolutely correct. And that's the reason it's giving you a compiler error. That being said, you'll need to take another look at the instructions to pass the challenge. It's not asking you to print anything but rather return the strings.
Good luck!
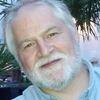
Jeff Muday
Treehouse Moderator 28,720 PointsRemove the last return statement... it is causing your issue.
A better way to do this would be to declare a string at the beginning of the function which will be the action to take based on the score.
The if statement block covers all three cases. So we can set the action value there. And then finally, since you wanted the function to print the action, we do that in one statement. When I look to the earlier question, I see a requirement to RETURN a string, so in the very last line of the function we return action
func PlayHand(score int) string {
var action string
if score < 17 {
action = "hit me"
} else if score > 21 {
action = "bust"
} else {
action = "stand"
}
fmt.Println(action)
return action
}
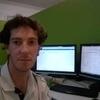
Gavin Mace
30,747 PointsThanks Jeff & Jennifer!
I took on both of those feedback points and solved it pretty much straight away.
I was also stuck on the Switch Statements challenge - for exactly the same reason. So your feedback helped me to tick off both of those challenges (Y).
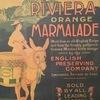
mehdilegoullon2
4,183 PointsI am blocked, need help please ! Bummer! Expected a score under 17 to return "hit me" but got "..." instead.
package blackjack
import "fmt"
func PlayHand(score int) string {
if score < 17 {
fmt.Println("hit me")
} else if score > 21 {
fmt.Println("bust")
} else {
fmt.Println("stand")
}
return "..."
}
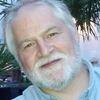
Jeff Muday
Treehouse Moderator 28,720 PointsTo assist, I would have to see more information on how PlayHand was called by the program. In addition, if you could reformat your code in a way I could see it all -- not all the code is inside the three backtick delimited blocks (see the Markdown cheatsheet). The reason why I stress this is GoLang is pickier than Java or C as to whitespace constraints.
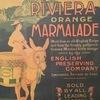
mehdilegoullon2
4,183 PointsI do not know what he asks me. And sorry for my English.
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherHi Jeff! I took the liberty of changing your comment to an answer to allow for voting and to mark the question as answered in the forums. Thanks for helping out in the Community!