Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial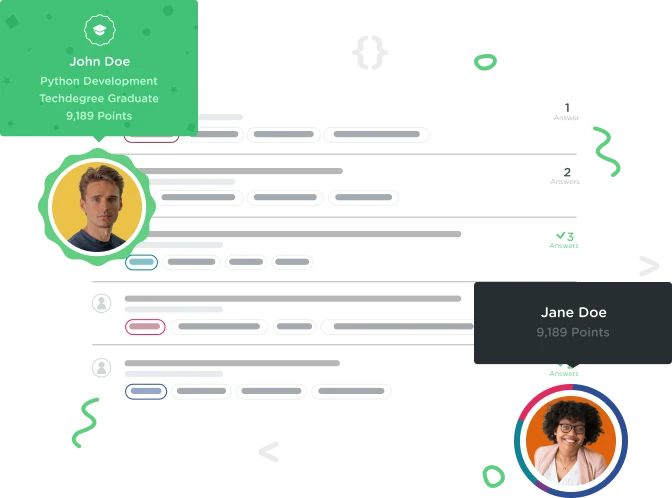
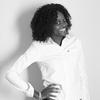
Nomzamo Sithole
61,838 PointsGo Lang - Last Task
What am i missing here?
package files
import (
"fmt"
"os"
)
func Size(fileName string) int64 {
fileInfo, err := os.Stat(fileName)
if err != nil {
// RETURN THE VALUES 0 AND err
fmt.Println(err)
}else {
fmt.Println(fileInfo.Size())
}
// RETURN THE VALUES fileInfo.Size() AND nil
fileInfo, error = os.Stat("nonexistent.txt")
if error != nil {
fmt.Println(error)
} else {
fmt.Println(fileInfo.Size())
}
}
2 Answers

Joe Purdy
23,237 PointsThe Size function currently returns just an
int64
value representing a file size. Update it so that it returns both thatint64
file size and anerror
value.
With Go, the function signature includes both the functions arguments and return value types. For example the function signature in this challenge takes a single argument of type string
and returns a single value of type int64
:
func Size(fileName string) int64 {}
Here's an example of a function signature that returns two types, first an int64
and also an error
:
func Foo(name string) (int64, error) {}
The first step for this challenge is to update the function signature of the Size function to return two values, an int64
and an error
, like in the example Foo function I listed above.
If the
err
return value fromos.Stat
is notnil
, thenSize
should return a file size of0
and whatever error value is inerr
.
To return multiple values in a Go function you simply write multiple values following the return
keyword in a function. For example to return a an int64
and a string
together you would write a return statement like this:
return 5, "foobar"
The challenge is asking you to write a return statement where it says // RETURN THE VALUES 0 AND err
that returns both the number 0 and the error value assigned to the variable err
. Currently you've written fmt.Println(err)
here when instead you should be using a return statement that sends two values, the number 0 and err.
If the
err
value fromos.Stat
isnil
, thenSize
should return the result of callingfileInfo.Size()
, plus an error value ofnil
.
Lastly where the challenge says // RETURN THE VALUES fileInfo.Size() AND nil
you need to write another return statement that returns two values like before. This time the return statement needs to send fileInfo.Size()
as the first value and nil
as the second. Here's an example of a return statement that sends the result of calling a method and a nil for the error value similar to what this challenge requires:
return foobar.Length(), nil
My examples here aren't exact answers so you'll need to adapt this to correctly pass the challenge. I hope that helps point you in the right direction, if this is still unclear try rewatching the Multiple Return Values video and feel free to ask for more clarity on what is unclear.
Cheers!

Tim Williams
Courses Plus Student 9,267 PointsJoe, thank you for explaining this without giving the answer away, this helped me arrive to a simple solution on my own (the struggle is where the understanding comes!!!) this problem is a lot simpler if you break down what is being asked here. All thats needed is to pass multiple return values so the function should be structured to pass multiple, Lastly give the function exactly what its asking you to return.
Nomzamo Sithole
61,838 PointsNomzamo Sithole
61,838 PointsThank you Joe Purdy i passed it. You explained it so well, i even missed the clues the code actually gave me