Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial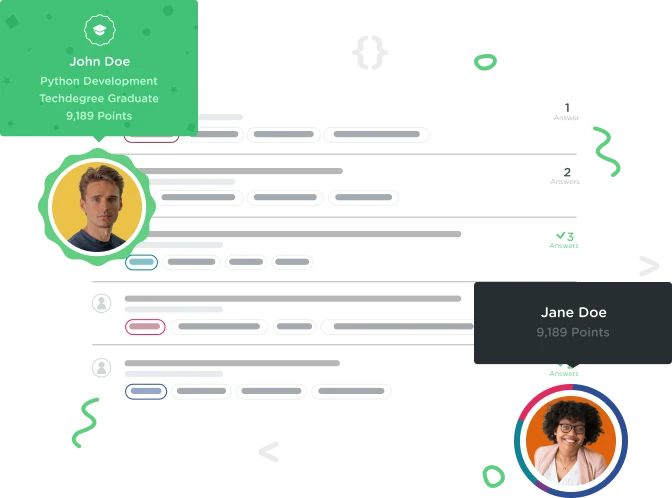

Nirbhay Agarwal
2,852 PointsGoKart
Error for get.Message()
class Example {
public static void main(String[] args) {
GoKart kart = new GoKart("purple");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
kart.drive(3);
kart.drive(1);
while(kart.charge()) {
System.out.println("Driven On");
}
try {
kart.drive(42);
System.out.println("Battery Down");
}catch (IllegalArgumentException iae) {
System.out.println("Whoa there");
System.out.printf("The error was %s \n",
iae.getMessage());
}
}
}
class GoKart {
public static final int MAX_BARS = 8;
private int barCount;
private String color;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
int newLaps = laps - barCount;
if (newLaps < barCount) {
throw new IllegalArgumentException("Not enough battery remain");
}
newLaps = barCount;
}
}
2 Answers

Unsubscribed User
1,785 PointsHey Nirbhay Agarwal!
First of all, in line ten of your first code block, you are trying to get a boolean back but in GoKart.java you defined the method .charge() to give NOTHING back (void). You would either have to change this:
public void charge() {
barCount = MAX_BARS;
}
to this:
public boolean charge() {
barCount = MAX_BARS;
return false;
}
But this wouldn't let the program print the message "Drive on" because a while-loop only runs while the condition is true. If .charge() returns FALSE now after having loaded barCount, the while-loop will automatically quit the loop and it will not print "Drive on".
You should put the code above into the if-statement where it is checking whether the battery is empty. Like this:
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
kart.charge();
System.out.println("Driven On");
}
I think the other problem you had was that you used "<" instead of ">" in this code:
public void drive(int laps) {
int newLaps = laps - barCount;
//RIGHT HERE//
if (newLaps < barCount) {
throw new IllegalArgumentException("Not enough battery remain");
}
newLaps = barCount;
}
I hope this helps! Julian

Seth Kroger
56,413 PointsIn this challenge you shouldn't have to alter the GoKart code at all (and I think the challenge ignores any edits to that file anyway). Try restarting from scratch. The only thing you need to do is put a try/catch block around the drive(42)
.
Nirbhay Agarwal
2,852 PointsNirbhay Agarwal
2,852 PointsIf I use > sign it means I can run more laps and it wont throw IllegalArgumentException
And then newlaps is != barCount.