Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial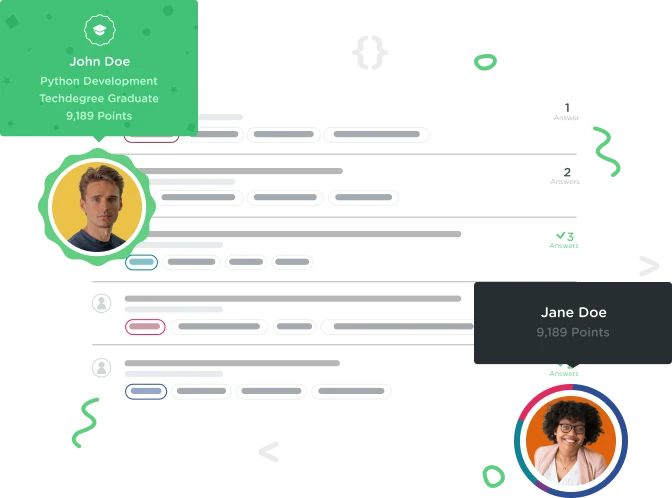
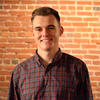
Henry Jackson
9,579 PointsGolang code example is not valid
func linearsearch(data []int, key int) bool {
for idx, item := range data {
if item == key {
return idx
}
}
return nil
}
This code would not be able to compile as the return value expected is a bool, but the function returns either an int or nil. The following would be valid:
func linearsearch(data []int, key int) bool {
for _, item := range data {
if item == key {
return true
}
}
return false
}
You may want to update the example for anyone else looking to write in Go!
1 Answer
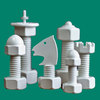
Steven Parker
241,809 PointsYou're right about the errors, and your suggested code would run just fine. But the purpose of the function is to return the index of the found item and a bool does not handle this.
So I would suggest having the function return an "int" value and using -1 to indicate "not found" as is done for some of the other language versions:
func linearsearch(data []int, key int) int {
for idx, item := range data {
if item == key {
return idx
}
}
return -1
}
I'm also passing this along to the staff in a bug report, hopefully it will be fixed soon.
Steven Parker
241,809 PointsSteven Parker
241,809 PointsPasan Premaratne — I'm also tagging you in case you'd like to look into this yourself.
Pasan Premaratne
Treehouse TeacherPasan Premaratne
Treehouse TeacherThanks folks! Go is not my area of expertise so I'll poke over this and update the docs as needed :)