Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial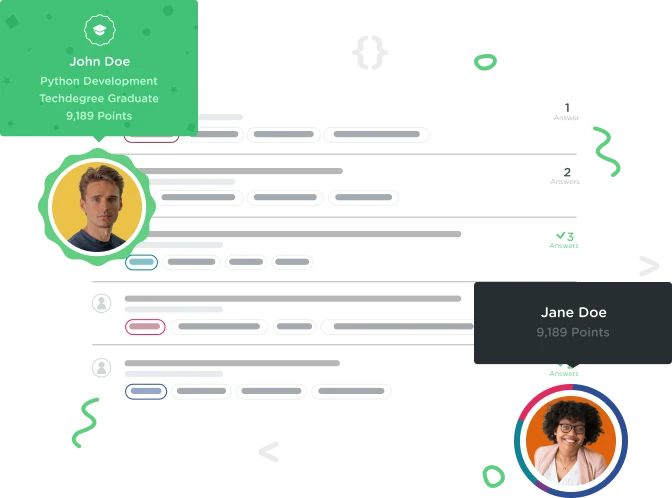

Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsGood day, i need some hints and suggestion regarding a project i am working on your help is very much appreciated.
/***
-
quotes
array- Create a variable named quotes and set it equal to an empty array.
- Add a minimum of five empty objects to your quotes array.
- Add data to your quote objects The objects in the quotes array store the individual properties of the quotes.
- Add the following properties to each quote object:
- quote - string - the actual quote
- source - string - the person or character who said it
- Add a citation property to at least one quote object. The value should be a string holding a reference to the source of the quote, like the book, movie or song where the quote originates.
- Add a year property to at least one quote object. The value should be a string or number representing the year the quote originated. ***/
/***
-
getRandomQuote
function 5.Create the getRandomQuote function The getRandomQuote function should create a random number, and use that random number to return a random quote object from the quotes array.- Create a function named getRandomQuote.
- In the function body, create a variable to store a random number ranging from zero to the index of the last item in the quotes array.
- Lastly, the function should return a random quote object using the random number variable above and bracket notation on the quotes array. ***/
/***
-
printQuote
function- Create the printQuote function The app should display a new quote each time the user clicks the "Show another quote" button using a printQuote function.
- Create a function named printQuote. You will program the printQuote function to perform three tasks: call the getRandomQuote function, use the returned quote object to build a string of HTML and quote properties, then use that string to display a random quote in the browser.
- In the body of the printQuote function, create a variable to store a random quote object from the getRandomQuote() function.
- Create another variable to store the HTML string. Set it equal to a string containing two <p> elements. Use this code snippet as a guide for what the HTML string should look like at this point: <p class="quote"> A random quote </p>
- <p class="source"> quote source </p>
- The first <p> element should have a class equal to “quote”, and the random quote object’s .quote property nested between the opening and closing <p> tags.
- The second <p> element should have a class equal to “source”, and the random quote object’s .source property nested between the tags.
- Create two separate if statements below the variables. You will need to add decision making to this function:
- Project Warm Up: Using conditionals to test if objects or elements exist before trying to do something with them is a common developer task. For some helpful practice, check out the project Warm Up Conditional String.
- If the random quote object has a citation property, concatenate a <span> element with the class "citation" to the HTML string.
- If the random quote object has a year property, concatenate a <span> element with the class "year" to the HTML string.
- Use the following code snippet as a guide for what the HTML string should look like with the added "citation" and "year" <span> elements (like earlier, omit the second closing </p> tag for now): <p class="quote"> A random quote </p>
- <p class="source"> quote source
- <span class="citation"> quote citation </span>
- <span class="year"> quote year </span>
- </p>
- Below the if statements, complete the string by concatenating a closing </p> tag to the HTML string. This is the closing tag for the second paragraph with the class source.
- Lastly, set the printQuote function to return the full HTML string displaying a random quote. Use the following code snippet, along with the variable storing the string you’ve assembled, to update your project’s HTML with a random quote: document.getElementById('quote-box').innerHTML = yourStringHere;
- Note: You'll learn all about document.getElementById() and .innerHTML in an upcoming course. In this project, it's going to help you select the 'quote-box' div and update its HTML content with the random quote markup.
***/
/***
- click event listener for the print quote button
- DO NOT CHANGE THE CODE BELOW!! ***/
document.getElementById('load-quote').addEventListener("click", printQuote, false);
Question: how do i code this? any hints and suggestions are welcome, thank you in advance for your help.
1 Answer
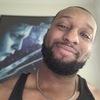
Brandon White
Full Stack JavaScript Techdegree Graduate 34,662 PointsHi Karl,
It appears that you're going through the a Tech Degree Program, or at the very least you're attempting one of the projects. A better forum to ask this question would be the Slack group for your Tech Degree. The people there are great, and many are working on the same project as you.
That said, a question like "How do I code this?" with respect to an entire app is perhaps a bit too broad. Why don't you start somewhere and when you run into a problem that you absolutely can't solve, then request help with that particular issue (while providing a link to the code you've already written).
If you don't feel like you even know where to start, I'd suggest reviewing the material for the unit you're working on a few more times.
Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsKarl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsGood day,
thank you for your response Mr.Brandon White, i understand you. that is why when i saw your post i made it already, but it does not seem to display anything, please see link of my workspace as follows: https://teamtreehouse.com/workspaces/41756901#
Things i did do: i only made script.js Things i did not do: i did not edit index.html, normalize.css and style.css.
My question do i need to edit them and how? any hints and suggestions is much appreciated, because the harvey specter quotes are not showing in the preview.
Again apologies for the previews post.