Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial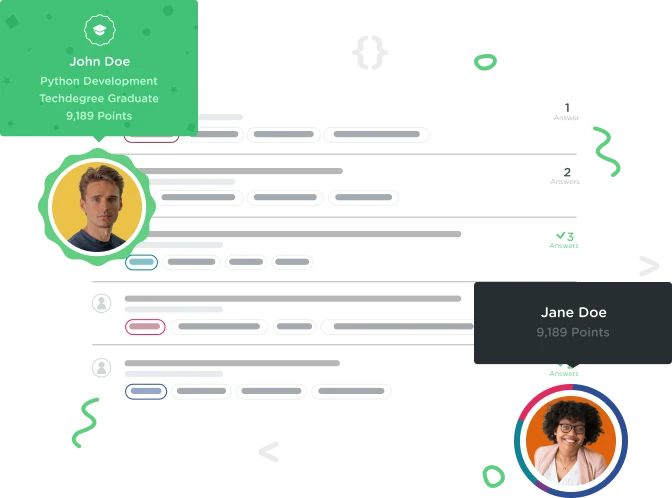

Johannes Ragnarsson
1,166 PointsGood praxis in try-block
Hi! New to this but it's really fun and challenging to try for yourself.
So, I solved the challenge with handling errors in a friendly manner but, I didn't use the solution as Craig Dennis did.
While Craig used the "if" and "raise" functions(?) within the try-block and ValueError to keep the User from entering a larger number than "tickets_remaining", my code handles the User-error after the try-block as seen below. After the User has entered an integer the code continues just as Craigs but in another way.
I want to know if what I've created is a "work-around" instead of handling the issue in the try-block or if I'm the right track but just need to tweak it a bit?
Please bring me some feedback on this :)
import math
TICKET_PRICE = 10
tickets_remaining = 100
while tickets_remaining >= 1:
print("There is right now {} tickets remaining to this show.".format(tickets_remaining))
name = input("Enter your name to start purchasing session: ")
try:
number_of_tickets = int(input("Hi {}! How many tickets would you like to buy? ".format(name)))
# Expect ValueError to happen and handle it appropriately... remember to test it out!
except ValueError:
print("Whoops, we ran into an issue with that value. Please try again.")
else:
#Here comes the handling the issue with number_of_tickets is larger than tickets_remaining
while number_of_tickets > tickets_remaining:
number_of_tickets = int(input("There are only {} tickets remaining, please enter a lower amount of tickets: ".format(tickets_remaining)))
ticket_total = number_of_tickets * TICKET_PRICE
print("That would be a total cost of ${}".format(ticket_total))
proceed = input("Would you like to proceed with the purchase?\n(Y/N)" )
if proceed.lower() == "y":
# TODO: Gather credit card information and process it.
print("SOLD!")
tickets_remaining -= number_of_tickets
print("({} tickets remaining)".format(tickets_remaining))
else:
print("Thank you {}. Until next time!".format(name))
else:
print("We are sorry but we are out of tickets")
1 Answer
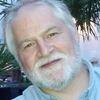
Jeff Muday
Treehouse Moderator 28,720 PointsIt is a good practice to show your code to others, nice job!
As far as "Best Practices" we never want to become "that guy that programs by Cut-and-Paste, Caveat, or Side-effect". And your code checks out well for the assignment. You use a ValueError
on the except
, that is great. Again it's too tempting to just except
all errors as equal-- resist that!
I tend to be a little more verbose in writing comments, you do a little better. I like to err on the side of explaining the code as I write it because, in six months or a year from now, you might ask "what was I thinking when I wrote this code" and you will know from the comments.
In the future, you will be taught by Craig, Kevin Love, or Jennifer Nordell to use the DRY principle when programming. "Don't Repeat Yourself". You're going to recognize code patterns where it makes sense to use functions/methods to encapsulate your code. That comes with time, you recognize good code when you see it.
Enjoy your Python journey!
Johannes Ragnarsson
1,166 PointsJohannes Ragnarsson
1,166 PointsThank you!