Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial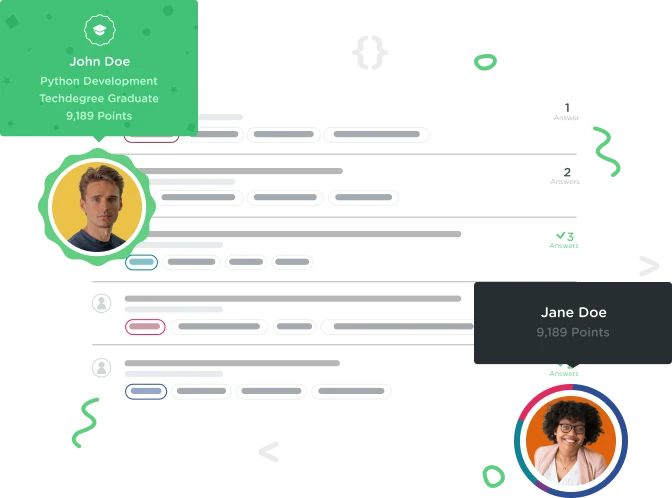
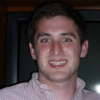
Richard Luick
10,955 PointsGoogle Search Extra Credit Help
Hello, so I have been moving along with the Google Search extra credit for "Getting Data from the Web" under the Building a Blog Reader project and have run into a little problem. I am currently working on saving the results the user has typed into an edit text view when a button is clicked so that I can use that input to search Google. I am able to get the button to log the text the user has typed inside the onClick method however, a log placed outside the method does not pick up the variable. I can only imagine this is some sort of scope issue but I do not understand since I have defined the variables outside the method. Here is the section of code with the listener.
private int connectToServer() throws MalformedURLException, IOException, JSONException {
int responseCode;
mSearchGoogleButton.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
mUserInput = mSearchText.getText().toString();
Log.v("SearchTextInside", mUserInput);
mSearchText.setText("");
}
});
Log.v("SearchTextOutside", mUserInput);
URL blogFeedUrl = new URL("https://ajax.googleapis.com/ajax/services/search/web?v=1.0&q=" + mUserInput);
HttpURLConnection connection = (HttpURLConnection) blogFeedUrl.openConnection();
connection.connect();
responseCode = connection.getResponseCode();
responseCodeCheck(responseCode, connection);
return responseCode;
}
And here is the entire program. Relevant variables are declared near the top.
package com.example.googlereaderec;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.app.ListActivity;
import android.content.Context;
import android.content.res.Resources;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
import android.os.AsyncTask;
import android.os.Bundle;
import android.util.Log;
import android.view.Menu;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainListActivity extends ListActivity {
protected String[] mBlogPostTitles;
protected String mException;
public static final int NUMBER_OF_POSTS = 20;
public static final String TAG = MainListActivity.class.getSimpleName();
Button mSearchGoogleButton;
EditText mSearchText;
String mUserInput = "";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main_list);
mSearchGoogleButton = (Button) findViewById(R.id.searchButton);
mSearchText = (EditText) findViewById(R.id.searchQuery);
Resources resources = getResources();
mException = resources.getString(R.string.exception);
if(isNetworkAvailable()) {
GetBlogPostsTask getBlogPostsTask = new GetBlogPostsTask();
getBlogPostsTask.execute();
}
else {
Toast.makeText(this, "Network is unavailable", Toast.LENGTH_LONG).show();
}
//Toast.makeText(this, getString(R.string.no_items), Toast.LENGTH_LONG).show();
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if(networkInfo != null && networkInfo.isConnected()) {
isAvailable = true;
}
return isAvailable;
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main_list, menu);
return true;
}
public class GetBlogPostsTask extends AsyncTask<Object, Void, String> {
@Override
protected String doInBackground(Object... params) {
int responseCode = -1;
try {
responseCode = connectToServer();
}
catch(MalformedURLException e) {
Log.e(TAG, mException, e);
}
catch(IOException e) {
Log.e(TAG, mException, e);
}
catch(Exception e) {
Log.e(TAG, mException, e);
}
return "Code: " + responseCode;
}
//Attempts to connect to the google API search result server based on the users input
private int connectToServer() throws MalformedURLException, IOException, JSONException {
int responseCode;
mSearchGoogleButton.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
mUserInput = mSearchText.getText().toString();
Log.v("SearchTextInside", mUserInput);
mSearchText.setText("");
}
});
Log.v("SearchTextOutside", mUserInput);
URL blogFeedUrl = new URL("https://ajax.googleapis.com/ajax/services/search/web?v=1.0&q=" + mUserInput);
HttpURLConnection connection = (HttpURLConnection) blogFeedUrl.openConnection();
connection.connect();
responseCode = connection.getResponseCode();
responseCodeCheck(responseCode, connection);
return responseCode;
}
//Checks if the response code is valid. If so, it executes the the code to get JSON results. If not, it logs an error
private void responseCodeCheck(int responseCode, HttpURLConnection connection) throws IOException, JSONException {
if(responseCode == HttpURLConnection.HTTP_OK) {
InputStream inputStream = connection.getInputStream();
Reader reader = new InputStreamReader(inputStream);
getJSONResults(reader);
}
else {
Log.i(TAG, "Unsuccessful HTTP Response Code: " + responseCode);
}
}
//Reads the JSON results into a string and extracts the url/other data from each result
private void getJSONResults(Reader reader) throws IOException, JSONException {
int nextCharacter; // read() returns an int, we cast it to char later
String responseData = "";
while(true){ // Infinite loop, can only be stopped by a "break" statement
nextCharacter = reader.read(); // read() without parameters returns one character
if(nextCharacter == -1) // A return value of -1 means that we reached the end
break;
responseData += (char) nextCharacter; // The += operator appends the character to the end of the string
}
JSONObject jsonResponse = new JSONObject(responseData);
JSONObject jsonResponseData = jsonResponse.getJSONObject("responseData");
JSONArray jsonResults = jsonResponseData.getJSONArray("results");
for(int i = 0; i < jsonResults.length(); i++) {
JSONObject jsonPost = jsonResults.getJSONObject(i);
String url = jsonPost.getString("url");
Log.v(TAG, "Result " + i + ": " + url);
}
}
}
}