Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial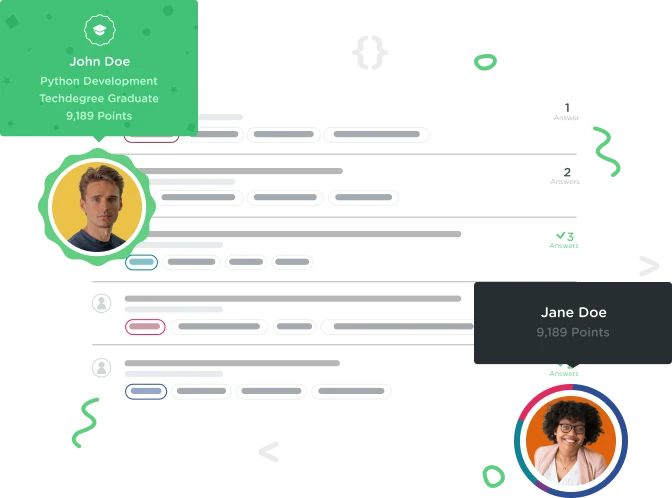

Yoel Dovid Cohen
13,405 Pointsgot confused
Can anyone help me! I started C#, then had to stop it for a few weeks and now I am stuck on this challenge and I don't know the answer to the question and I forgot what is a parameter and what is a constructor. Also where is the video that deals with it, as I can't listen to all of the videos again. A big thank you.
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
public bool EatFly(int distanceToFly)
{
return TongueLength >= distanceToFly;
}
public readonly int ReactionTime;
public int reactionTime(ReactionTime)
{
reactionTime = ReactionTime;
return int reactionTime(ReactionTime);
}
}
}
1 Answer
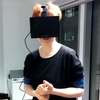
Mia Filisch
16,117 PointsHey! A parameter is something that defines what arguments you can pass into a method when calling it, so in C# that's the bits in parenthesis, for example int distanceToFly
in this line:
public bool EatFly(int distanceToFly)
A constructor in C# is a special type of method that is used to make a new instance (a new object) of a given class. Like other methods, it can also take parameters. You can always identify the constructor by looking for the thing that has the same name as the class (unlike normal methods, it will also have no void or return type stated as this isn't applicable to a constructor).
So in this example, the constructor is:
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
And therefore the solution would be:
public Frog(int tongueLength, int reactionTime)
{
TongueLength = tongueLength;
ReactionTime = reactionTime;
}
If it is still confusing, it might make sense to re-watch the videos as this is quite important stuff to get your head around it, you'll use it all the time in C#!