Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial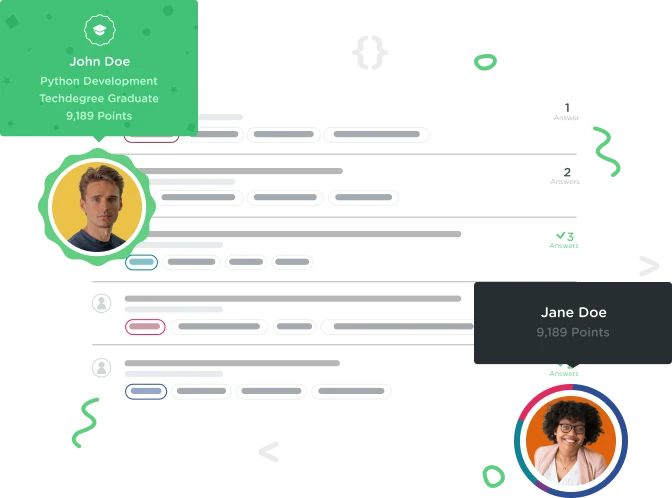

Dillon Reyna
9,531 PointsGot the wrong string for reversed?
Here's the code I used - checked in repl to ensure that it reversed the given string completely. What's missing?
def stringcases(x):
tlist = []
a = x.upper()
b = x.lower()
c = x.title()
for char in x:
tlist.append(char)
d = tlist[::-1]
return(a, b, c, d)
1 Answer

andren
28,558 PointsThe issue is that you return the string as a reversed list, not a reversed string. Since you convert it into a list you have to convert it back into a string again before you return it.
You can convert it to a string by joining the list like this:
def stringcases(x):
tlist = []
a = x.upper()
b = x.lower()
c = x.title()
for char in x:
tlist.append(char)
d = ''.join(tlist[::-1])
return(a, b, c, d)
However you didn't really need to convert it to a list in the first place, as slices work on strings just like they do on lists. That means that you can slice the string directly like this:
def stringcases(x):
a = x.upper()
b = x.lower()
c = x.title()
d = x[::-1]
return(a, b, c, d)
Which is a lot simpler than converting it to a list.