Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial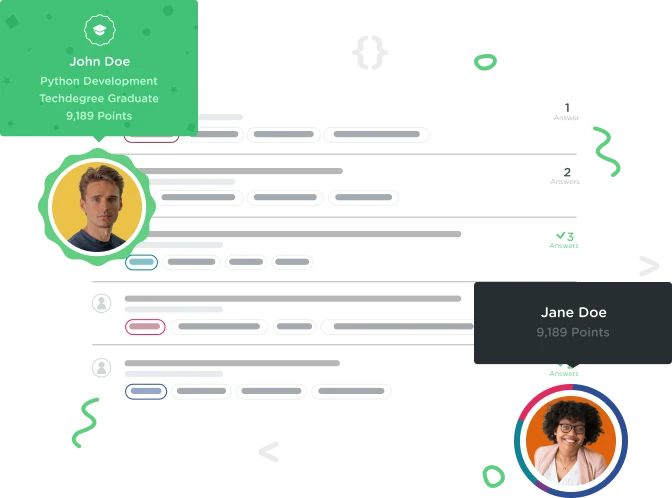

Jennifer Chance
Courses Plus Student 1,424 PointsGradle error when i try to run my app. Any help would be appreciated. I have been following the tutorial code for code.
Getting a Gradle error when i run my app. I have red line errors on blogPosts in main source code.
Here is my source code:
package com.jerdeez.blogreader;
import android.app.AlertDialog;
import android.app.ListActivity;
import android.content.Context;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
import android.os.AsyncTask;
import android.os.Bundle;
import android.text.Html;
import android.util.Log;
import android.view.Menu;
import android.view.View;
import android.widget.ArrayAdapter;
import android.widget.ProgressBar;
import android.widget.SimpleAdapter;
import android.widget.TextView;
import android.widget.Toast;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.ArrayList;
import java.util.HashMap;
public class MainListActivity extends ListActivity {
public static final int NUMBER_OF_POSTS = 20;
public static final String TAG = MainListActivity.class.getSimpleName();
protected JSONObject mBlogData;
protected ProgressBar mProgressBar;
private final String KEY_TITLE ="title";
private final String KEY_AUTHOR ="author";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main_list);
mProgressBar = (ProgressBar)findViewById(R.id.progressBar1);
if (isNetworkAvailable()) {
mProgressBar.setVisibility(View.VISIBLE);
GetBlogPostsTask getBlogPostsTask = new GetBlogPostsTask();
getBlogPostsTask.execute();
//Toast.makeText(this, getString(R.string.no_items),Toast.LENGTH_LONG).show();
} else {
Toast.makeText(this, "Network is unavailable!", Toast.LENGTH_LONG).show();
}
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager)
getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected()) {
isAvailable = true;
}
return isAvailable;
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main_list, menu);
return true;
}
public void handleBlogresponse() {
mProgressBar.setVisibility(View.INVISIBLE);
if (mBlogData == null) {
updateDisplayForError();
}
else{
try {
JSONArray jsonPost = mBlogData.getJSONArray("posts");
//JSONArray jsonPost = mBlogData.getJSONArray("post");
ArrayList<HashMap<String, String>> blogPost = new ArrayList<HashMap<String, String>>();
for (int i =0; i<jsonPost.length(); i++){
JSONObject post = jsonPost.getJSONObject(i);
String title = post.getString(KEY_TITLE);
title = Html.fromHtml(title).toString();
String author = post.getString(KEY_AUTHOR);
author = Html.fromHtml(author).toString();
HashMap<String, String> blogPost = new HashMap<String, String>();
blogPost.put(KEY_TITLE, title);
blogPost.put(KEY_AUTHOR, author);
blogPosts.add(blogPost);
}
String [] keys = {KEY_TITLE, KEY_AUTHOR};
int [] ids ={android.R.id.text1, android.R.id.text2};
SimpleAdapter adapter = new SimpleAdapter(this, blogPosts,
android.R.layout.simple_list_item_2, keys, ids);
setListAdapter(adapter);
}
catch (JSONException e) {
Log.e(TAG, "Exception Caught!", e);
}
}
}
private void updateDisplayForError() {
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle(getString(R.string.error_title));
builder.setMessage(getString(R.string.error_message));
builder.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
TextView emptyTextView = (TextView) getListView().getEmptyView();
emptyTextView.setText(getString(R.string.no_items));
}
private class GetBlogPostsTask extends AsyncTask<Object, Void, JSONObject> {
@Override
protected JSONObject doInBackground(Object[] objects) {
int responseCode = -1;
JSONObject jsonResponse = null;
try {
//URL blogFeedUrl = new URL("http://blog.teamtreehouse.com/api/get_recent_summary/?count =" + NUMBER_OF_POSTS);
URL blogFeedUrl = new URL("http://blog.teamtreehouse.com/api/get_recent_summary/?count=" + NUMBER_OF_POSTS);
HttpURLConnection connection = (HttpURLConnection) blogFeedUrl.openConnection();
connection.connect();
//Creating are response code so that we can get data from the internet
responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
InputStream inputStream = connection.getInputStream(); //store the data into the input stream
Reader reader = new InputStreamReader(inputStream);//read the input stream
int contentLength = connection.getContentLength();//get the number of characters to read in
char[] charArray = new char[contentLength];//create the char array to store the the data
reader.read(charArray); //read and store the data array into the char array
String responseData = new String(charArray);//create a new string and convert and store from char to string
//This creates are Json object
jsonResponse = new JSONObject(responseData);
}
//} else {
//Log.i(TAG, "Unsuccessful HTTP Response Code: " + responseCode);
//}
Log.i(TAG, "Code: " + responseCode);
} catch (MalformedURLException e) {
//e.printStackTrace();
Log.e(TAG, "Exception caught: ", e);
} catch (IOException e) {
Log.e(TAG, "Exception caught: ", e);
} catch (Exception e) {
Log.e(TAG, "Exception caught: ", e);
}
return jsonResponse;
}
@Override
protected void onPostExecute(JSONObject result) {
mBlogData = result;
handleBlogresponse();
}
}
}
Here is my Gradle build error:
Total time: 6.461 secs
Executing tasks: [:app:assembleDebug]
Configuration on demand is an incubating feature.
Relying on packaging to define the extension of the main artifact has been deprecated and is scheduled to be removed in Gradle 2.0
:app:preBuild
:app:compileDebugNdk UP-TO-DATE
:app:preDebugBuild
:app:checkDebugManifest
:app:preReleaseBuild
:app:prepareComAndroidSupportAppcompatV72000Library UP-TO-DATE
:app:prepareComAndroidSupportSupportV42000Library UP-TO-DATE
:app:prepareDebugDependencies
:app:compileDebugAidl UP-TO-DATE
:app:compileDebugRenderscript UP-TO-DATE
:app:generateDebugBuildConfig UP-TO-DATE
:app:generateDebugAssets UP-TO-DATE
:app:mergeDebugAssets UP-TO-DATE
:app:generateDebugResValues UP-TO-DATE
:app:generateDebugResources UP-TO-DATE
:app:mergeDebugResources UP-TO-DATE
:app:processDebugManifest UP-TO-DATE
:app:processDebugResources UP-TO-DATE
:app:generateDebugSources UP-TO-DATE
:app:compileDebugJava
C:\Users\Owner-1\AndroidStudioProjects\BlogReader\app\src\main\java\com\jerdeez\blogreader\MainListActivity.java:104: error: variable blogPost is already defined in method handleBlogresponse()
HashMap<String, String> blogPost = new HashMap<String, String>();
^
C:\Users\Owner-1\AndroidStudioProjects\BlogReader\app\src\main\java\com\jerdeez\blogreader\MainListActivity.java:109: error: cannot find symbol
blogPosts.add(blogPost);
^
symbol: variable blogPosts
location: class MainListActivity
C:\Users\Owner-1\AndroidStudioProjects\BlogReader\app\src\main\java\com\jerdeez\blogreader\MainListActivity.java:114: error: cannot find symbol
SimpleAdapter adapter = new SimpleAdapter(this, blogPosts,
^
symbol: variable blogPosts
location: class MainListActivity
3 errors
FAILED
FAILURE: Build failed with an exception.
* What went wrong:
Execution failed for task ':app:compileDebugJava'.
> Compilation failed; see the compiler error output for details.
* Try:
Run with --stacktrace option to get the stack trace. Run with --info or --debug option to get more log output.
BUILD FAILED
Total time: 3.896 secs
1 Answer

william parrish
13,774 PointsIn your handle blog response method, you define both an ArrayList, and a Hashmap as the same variable, named blogpost.
ArrayList<HashMap<String, String>> blogPost = new ArrayList<HashMap<String, String>>();
HashMap<String, String> blogPost = new HashMap<String, String>();
You then try to add something to "blogposts" but blog posts doesnt exist yet. Likely just a simple oversight, and you forgot to add an S to one of these.
What is amazing about this though, is your gradle error clearly defines the problem for you, and tells you where to go to fix it. Check it out :
C:\Users\Owner-1\AndroidStudioProjects\BlogReader\app\src\main\java\com\jerdeez\blogreader\MainListActivity.java:104: error: variable blogPost is already defined in method handleBlogresponse() HashMap<String, String> blogPost = new HashMap<String, String>();
The '104' is the line number that will let you go right to where you did something wrong, and gradle is really good about telling you where you went wrong. Dont overlook that information. I know it looks like a pile of crazy lines, but take the time to find the important ones, they stick out when you know what to look for.
Jennifer Chance
Courses Plus Student 1,424 PointsJennifer Chance
Courses Plus Student 1,424 PointsWilliam,
I overlooked the error message. I fixed the problem like suggested i had blogpost instead of blogposts. Thanks for your help!