Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial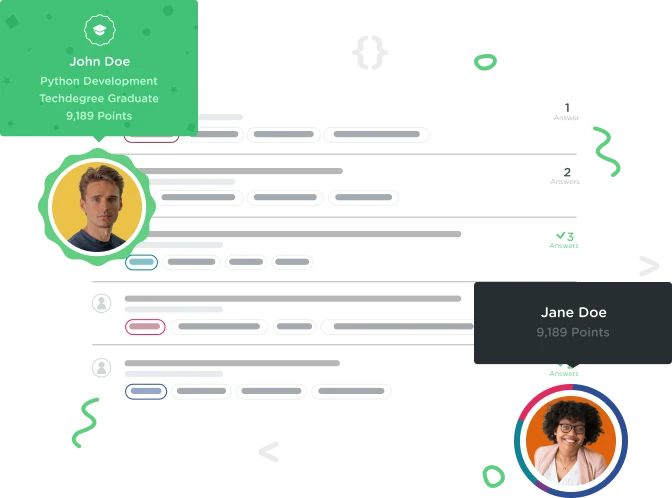

Kyle Melton
6,664 PointsGrand Central Dispatch, Say Whaa
I need help with using the GCD. I am trying to fetch body weight and body fat from the Health Kit and multiply them together then list that out in a table view to the user.
Here's how I think it's supposed to be set up.
In my controller Instantiate the model object -> Model object makes an async call to the Health Kit for body weight in a dispatch group -> Model object makes another async call in the same dispatch group for body fat -> the group finishes and notifies the controller -> on the main dispatch queue I access the now fully instantiated model object to get the data -> insert rows into the table with the data
How do I notify the controller that the DispatchGroup in the model has completed?
Please let me know what code I need to provide to assist in answering. I have a lot of code and didn't want to paste it all.
Any help is much appreciated.
2 Answers

Martin Wildfeuer
Courses Plus Student 11,071 PointsHey Kyle Melton,
I have added a playground example that hopefully somehow matches your use case. It is partly taken from AllAboutSwift. I tend to not post cross links here, but it is a huge topic and I think it would be too much to explain it here.
Also, when it comes to async programming and queues, you might want to have look at Operation Queue.
Frameworks worth having a look at:
- PromiseKit
- RxSwift and plenty other reactive libraries
Please note that these might be overkill for what you are trying to achieve. I just want to highlight that, as async tasks are literally everywhere in an app, there are great libraries to help you here.
Finally, though, the code example
import UIKit
import PlaygroundSupport
PlaygroundPage.current.needsIndefiniteExecution = true
class Client {
let queue = DispatchQueue(label: "com.allaboutswift.dispatchgroup", attributes: .concurrent, target: .main)
let group = DispatchGroup()
func taskOne() {
queue.async(group: group) {
print("Waiting for task 1 to finish")
Thread.sleep(forTimeInterval: 1)
print("Task 1 finished")
}
}
func taskTwo() {
queue.async(group: group) {
print("Waiting for task 2 to finish")
Thread.sleep(forTimeInterval: 1)
print("Task 2 finished")
}
}
func taskOneAndTwo() {
taskOne()
taskTwo()
group.notify(queue: .main) {
print("Task 1 & 2 finished")
}
}
}
let client = Client()
client.taskOneAndTwo()
Hope that helps :)

Kyle Melton
6,664 PointsThank you! Yes it does help. I would love a course that goes through incorporating asynchronous programming with Swift 3. Most of the resources available are outdated.