Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial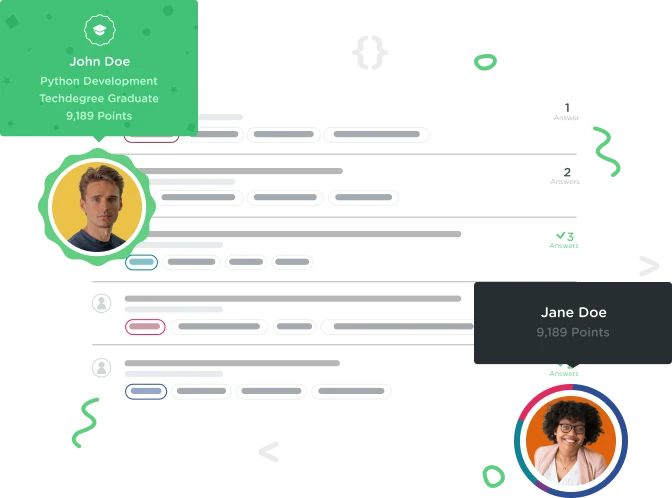

kok shhs
88 PointsGreat! Now use .remove() and/or del to remove the string, the boolean, and the list from inside of messy_list. When you'
How do i delete the list in the list ?!
messy_list = ["a", 2, 3, 1, False, [1, 2, 3]]
messy_list.pop(3)
messy_list.insert(0, 1)
messy_list.remove("a")
messy_list.remove(False)
del messy_list[5,6,7]
4 Answers
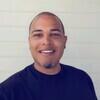
Eric Fernandez
Python Development Techdegree Student 3,008 PointsIs there a better way of doing this
if "a" in messy_list:
messy_list.remove("a")
if False in messy_list:
messy_list.remove(False)
if [1,2,3] in messy_list:
del messy_list[3]

f4u57
6,743 Pointsmessy_list.remove("a")
messy_list.remove(False)
messy_list.remove([1, 2, 3])

David Toops
19,153 PointsHere is a more general way to remove non-integer items from an arbitrary list:
for item in messy_list[::-1]: if isinstance(item,int): if isinstance(item,bool): messy_list.remove(item) else: messy_list.remove(item)

Tate Price
Courses Plus Student 9,987 Pointsmessy_list.remove([1, 2, 3])
hope that helps
Enzie Riddle
Front End Web Development Techdegree Graduate 19,278 PointsEnzie Riddle
Front End Web Development Techdegree Graduate 19,278 PointsAh you are so close! Remember that when you use the
del
keyword that you must use the item's index to delete it. Since you have already been using the remove method, I recommend you stick with it, but you definitely can complete it either way! Here's an example. Comment back if you're still having trouble!