Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial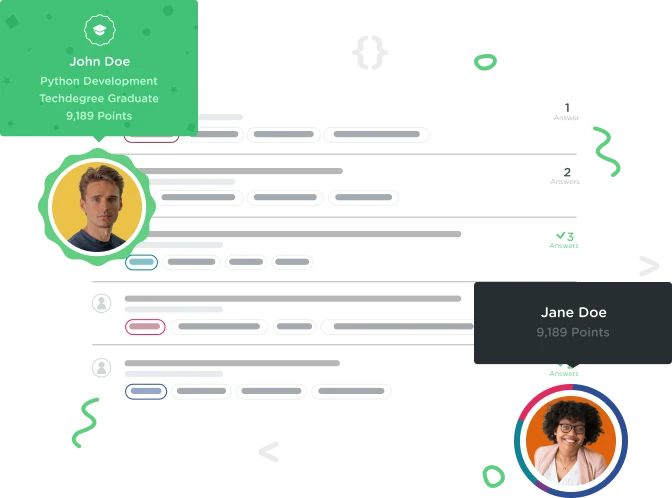
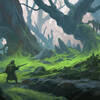
Caleb Newell
12,562 Pointsgroups.py
this is challenge task two, and i seem to be stuck. I'm trying to only run trios in the musical groups. Any help would be much thanked.
musical_groups = [
["Ad Rock", "MCA", "Mike D."],
["John Lennon", "Paul McCartney", "Ringo Starr", "George Harrison"],
["Salt", "Peppa", "Spinderella"],
["Rivers Cuomo", "Patrick Wilson", "Brian Bell", "Scott Shriner"],
["Chuck D.", "Flavor Flav", "Professor Griff", "Khari Winn", "DJ Lord"],
["Axl Rose", "Slash", "Duff McKagan", "Steven Adler"],
["Run", "DMC", "Jam Master Jay"],
]
# Your code here
for musical_group in musical_groups:
musical_group_line = ", ".join(musical_group)
print("musical groups: " + musical_group_line)
trios = [0, 2, 6]
for trios in musical_groups:
print(trios)
2 Answers
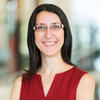
Louise St. Germain
19,424 PointsHi Caleb,
In the second part of the challenge, it's asking you to modify what you wrote in the first part of the challenge, so that it only prints the group (using the format you implemented in part 1) if it has 3 members. And if it doesn't have 3 members, don't print that group.
Here's some pseudo-code that should help:
for musical_group in musical_groups:
# TODO: add line of code that checks:
# if the number of members in that group is 3: (hint: use len() function)
musical_group_line = ", ".join(musical_group)
print("musical groups: " + musical_group_line)
# There's no "else" required here, since for everything else
# it's supposed to do nothing anyway.
You're already close, and the answer is simpler than you probably expect! :-)
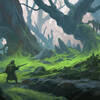
Caleb Newell
12,562 Pointsfor musical_group in musical_groups:
if musical_group == 3:
print(len([0, 2, 6]))
musical_group_line = ", ".join(musical_group)
print("musical groups: " + musical_group_line)
i tried this but it would not print the 3. Hmmm. :/ a little more help if you wouldn't mind.
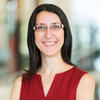
Louise St. Germain
19,424 PointsHi Caleb!
You literally just need to add an if statement, indent what you already have, and it's good to go. Note that the challenge wants you to actually print out the names in each trio (as you did when you printed the names of all band members before, regardless of number of people in the band).
for musical_group in musical_groups:
if len(musical_group) == 3:
musical_group_line = ", ".join(musical_group)
print("musical groups: " + musical_group_line)
When you use the for loop on musical_groups, it iterates through each item of the list. In this case, these are each another list. So the first time through musical_groups, your musical_group variable will get assigned the value ["Ad Rock", "MCA", "Mike D."]. The second time around, it will be ["John Lennon", "Paul McCartney", "Ringo Starr", "George Harrison"]. And so on until it gets to the end of the musical_groups list.
Since these within musical_groups are all lists themselves, you can use the len function on them (i.e., on musical_group) to get the length, which in this case, represents the number of band members.
The working code doesn't require you to manually identify which groups are trios in advance, which is what I think you were getting at with [0, 2, 6]. The way you had it set up isn't going to work because as I mentioned, each musical_group is a list of band members, so when you straight up compare it (= a list) to the integer "3", that will never be True, and so it will never print what's on the next line. (Which would only just print "3" because there are 3 items in the list [0, 2, 6] - Python has no idea you're trying to tell it index numbers.)
Also, in your latest example, since the last 2 lines are not indented within the if statement, it will always do them, so in the end, your new code works exactly the same as the code in part 1. So that's why they need to be indented, inside the if statement - so the printing only happens if there are 3 band members.
Anyway, I hope this helps a bit more! Let me know if you still have questions.

mohan Abdul
Courses Plus Student 1,453 Pointsi would have never have guessed to have used len, len function identifies how many words there are. how does this translate into len knowing which words contain 3 band members?
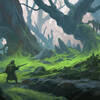
Caleb Newell
12,562 PointsThanks for the help :) It worked. now i know what i did wrong.
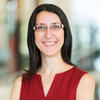
Louise St. Germain
19,424 PointsYou're welcome - glad you got it working! :-)
mohan Abdul
Courses Plus Student 1,453 Pointsmohan Abdul
Courses Plus Student 1,453 Pointsi would have never have guessed to have used len, len function identifies how many words there are. how does this translate into len knowing which words contain 3 band members?