Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial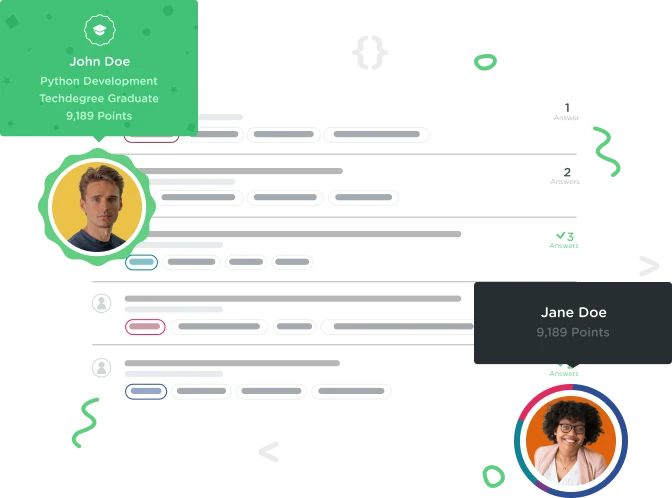

David Burks
731 Pointsguess game: My guess
So I decided to take Ken up on his challenge and am trying to write my own game code. But when I run my script, I keep getting errors regarding the indent. so far it has been on the line 2 of the code (where I have an 'if' in the function im defining.) Any ideas why? Also, you'll see a part in this code where I was making sure that the player was entering a number, so I wanted Python to verify that the player gave me an int. (Sorry, bad habit created by my Perl professor!)
def validate_guess():
if plyr_guess != int:
print("Whoops! You didn't enter a number!")
plyr_guess = input("Please enter a number between 1-10.")
elif plyr_guess > 10:
print("Whoops! You're number should be between 1-10.")
plyr_guess = input("Please enter a number between 1-10.")
else:
break
def count_guess():
while True:
count < 4:
if plyr_guess != target_num:
elif plyr_guess < target_num:
print("Nope! Guess higher!")
count += 1
elif plyr_guess > target_num:
print("Nope! Guess lower!")
count += 1
else:
print("Congratulations! You won in", count, " tries!")
exit()
import random
target_num = random.randint(1,10)
total = 0
count = 0
#Explain the rules of game
print("Hmmmm. . . . I'm thinking of a number between 1 and 10. Can you guess it? I'll give you 3 tries.)"
plyr_guess = input("Please enter a number between 1-10.")
random_num()
#Read guess and validate that plyr_guess is an integer.
validate_guess()
evaluate_num()

mrben
8,068 PointsSee this for details on posting code.

Geoff Parsons
11,679 PointsI added the markdown for formatting and syntax highlighting for you David Burks. Check out the post mrben pointed out for more info.
1 Answer

Geoff Parsons
11,679 PointsHere's a quick visual take on what i see that are likely causing some of your issues. I may have missed something or misinterpreted.
def validate_guess():
if plyr_guess != int:
print("Whoops! You didn't enter a number!")
plyr_guess = input("Please enter a number between 1-10.")
elif plyr_guess > 10:
print("Whoops! You're number should be between 1-10.")
plyr_guess = input("Please enter a number between 1-10.")
else:
break
- You'll need to indent the method body (everything after the
def
line). - You're using
break
here which is to break out of a loop (i.e. exit the loop early) and isn't valid or needed here. The method will return at the end of execution if neither of the two if / elif statements are true, so you can safely remove the else and break lines here.
def count_guess():
while True:
count < 4:
if plyr_guess != target_num:
elif plyr_guess < target_num:
print("Nope! Guess higher!")
count += 1
elif plyr_guess > target_num:
print("Nope! Guess lower!")
count += 1
else:
print("Congratulations! You won in", count, " tries!")
exit()
- Again you'll need to indent the method body.
- Was
count < 4
line meant to be the condition of the loop instead ofwhile True
? - I believe the first if condition is redundant as it will be covered by either
plyr_guess < target_num
orplyr_guess > target_num
and may also be a syntax error since it has no body. - Also i think the
else
line here is meant to be in line with the rest of the conditions? If not it will need a corresponding if statement.
import random
target_num = random.randint(1,10)
total = 0
count = 0
#Explain the rules of game
print("Hmmmm. . . . I'm thinking of a number between 1 and 10. Can you guess it? I'll give you 3 tries.)"
plyr_guess = input("Please enter a number between 1-10.")
random_num()
#Read guess and validate that plyr_guess is an integer.
validate_guess()
evaluate_num()
- It's customary to include import calls at the top of the file.
- Your closing parenthesis of the line that prints the game rules is inside of your quotes.
- You haven't defined
random_num()
Hope that helps get you on the way! Post the results when you're done so we can try it out!
Cheers.

silashunter
Courses Plus Student 162 PointsAnd I guess (yes hehe) this line won't work or won't work as it's expected:
if plyr_guess != int:
Because Kenneth told me something about you cannot compare a concrete value to whole class group like int is. He advised to use this thing instead:
isinstance(5, int)
It returns either True or False, so you have to manage output for it.
David Burks
731 PointsDavid Burks
731 PointsI am so sorry my code looks like this. It was fine until I clicked on the 'start discussion' tab. How do I get these to display right?