Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial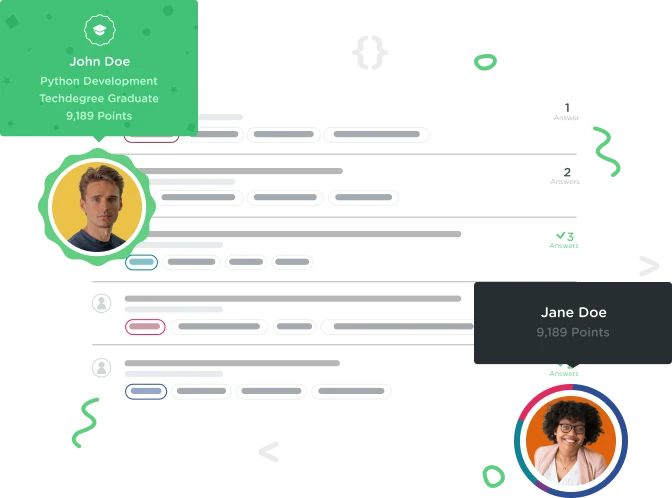

intrudir
1,664 PointsGuess word correctly but game will not end?
Hi guys, so I have been following along with the video for the letter game. So far everything happens as it should however if the full word has been guessed correctly it keeps asking for more guesses and i am totally lost. Please help!
import random
words = [
'apple',
'banana',
'orange',
'coconut',
'lemon',
'kumquat',
'grapefruit'
]
while True:
start = input("press Enter/return to start, or Q to quit: ")
if start.lower() == 'q':
break
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while len(bad_guesses) < 7 and len(good_guesses) != len(list(secret_word)):
for letter in secret_word:
if letter in good_guesses:
print(letter, end='')
else:
print('_', end='')
print('')
print('Strikes: {}/7'.format(len(bad_guesses)))
print('')
guess = input("Please enter your guess: ").lower()
if len(guess) != 1:
print("You can only guess a single letter!")
continue
elif guess in bad_guesses or guess in good_guesses:
print("You've already guessed that letter!")
continue
elif not guess.isalpha():
print("You can only guess letters!")
continue
if guess in secret_word:
good_guesses.append(guess)
if len(good_guesses) == len(list(secret_word)):
print("You win! The word was {}".format(secret_word))
break
else:
bad_guesses.append(guess)
else:
print("You didn't guess it! My secret word was {}".format(secret_word))
2 Answers
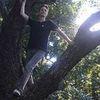
Gyorgy Andorka
13,811 PointsHi! Could you please edit your post so that your code is wrapped like this (for syntax highlighting and proper indentation): <three backticks + language name> <your code starting on a new line> <three backtics on a new line>? (I cannot type it because it would be automatically formatted; here is help on posting code with proper formatting: https://teamtreehouse.com/community/howto-guide-markdown-within-posts) EDIT: oh, I see that you tried that, but used '-s insted of backtics, hence the problem.
Anyway, I think I've found the bug: your program works e.g. for "orange", but not for "apple" or "coconut" - can you guess why? Here's the problem:
if len(good_guesses) == len(list(secret_word))
is not a good condition check, because it will work only if no letter appears more than once in the secret word. Otherwise, even if you guess all letters correctly, len(good_guesses)
will still be less than the length of the secret world, and the program will keep asking for another guess infinitely... Try to come up with a different condition check - at the bottom I included an elegant one :) *
(Also note that the second condition check in the (inner) while
loop was redundant, since you checked for the same thing inside the loop, and included a break
statement afterwards.)
- As promised, a short solution to the "guessed all" problem (using
set()
):
if set(good_guesses) == set(secret_word)
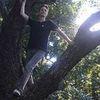
Gyorgy Andorka
13,811 Pointsset(<iterable>)
returns an unordered collection of unique elements (a set), so it's guaranteed that every item will appear only once. It's a common approach for removing duplicates from a list (then we use the list()
method to convert it back to list format):
my_list_without_duplicates = list(set(my_list))
Note that since sets are unordered, the original order of the list will be lost! It's a bit more tricky if you need to preserve the order - you can read more about that e.g. here: http://stackoverflow.com/questions/480214/how-do-you-remove-duplicates-from-a-list-in-whilst-preserving-order

intrudir
1,664 PointsExcellent, thank you so much!
intrudir
1,664 Pointsintrudir
1,664 PointsThanks for taking the time to help me out! I was able to figure out how to format the code for proper highlighting and such! As for my issue, the last else block is actually for the second while loop. I was following along with the video and thats how Kenny did it. Same goes for the if block len(good guesses) == len(list(secret_word)) i was trying to figure out why it worked for Kenny without issues. As my code is right now, i dont have any errors and the program runs in its entirety, minus the not getting a "You got it right!" statement
Gyorgy Andorka
13,811 PointsGyorgy Andorka
13,811 PointsOh, sorry, I completely forgot about the
while...else
syntax in Python, yeah, it's totally fine like that. (I've edited my post and removed that part.)Gyorgy Andorka
13,811 PointsGyorgy Andorka
13,811 PointsActually, Kenny's version was (intentionally) buggy :) Check the bottom of the page ("did you notice?"): https://teamtreehouse.com/library/python-basics/letter-game-app/letter-game-introduction
(I've just checked out, he will use - spoiler - a
for
loop and a boolean flag in the next video to solve that problem.)intrudir
1,664 Pointsintrudir
1,664 PointsOhhh okay. So that part about it endlessly asking for a letter when there is a recurring letter was an intentional bug? I understand now what you meant by using a different conditional check. I still don't know how to fix it but I will keep trying! Thank you sir!
PS. Can you elaborate more on what set does when used in an if statement?