Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial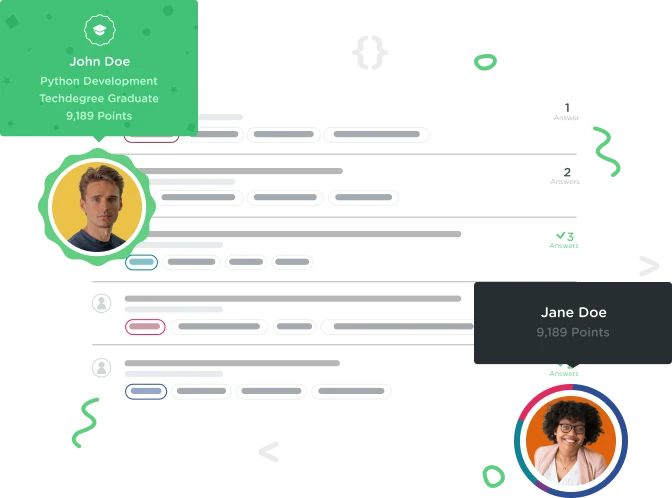

Christopher Borchardt
2,908 Pointsguessing game not running
I am getting a little ahead of the courses in the javascript basics. I am trying to combine what has been taught in the javascript course with other principles I've learned in other course.
I am trying to make a guessing game that the user can pick how high the number goes, and the number of guesses scales with how large the number is.
I have it set to loop for the number of guesses selected.
However when i try to run the code in a workspace it doesn't run.
/*
Random number guessing game.
The user can select a number and the game
Will generate a random number from 1 to the number selected.
The user has a different number of chances to guess the number
depending on how large a number selected
*/
// Prompt user to pick how high the random number will go and generate the random number
var input = prompt("Pick a number");
var randomNumber = Math.floor(Math.random()* input) + 1;
var guesses = 0;
// dermine the number of guesses allowed by how large a number the user picks.
function numberOfGuesses (input) {
if input <= 10{
guesses = 3 ;
} else if (input <= 100){
guesses = 8;
} else if (input <= 1000){
guesses = 15;
}else {
guesses = math.float(Number*.3);
}
}
// compare the guess to the random number generated.
function main (guesses) {
for( i = guesses, i >0, i--)
var guess = prompt('I am thinking of a number between 1 and ' + input + '. What is it? You can guess ' + guesses + 'time(s)');
if (parseInt(guess) === randomNumber) {
document.write('<p>you guessed the number!</p>');
} else if (guess < randomNumber) {
document.write('Your guess was too low, try again!');
guesses -= 1
} else {
document.write('Your guess was too high, try again!');
guesses -= 1
}
}
}
numberOfGuesses(input);
main(guesses);
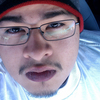
Chyno Deluxe
16,936 Points//Fixed Code Preview
1 Answer
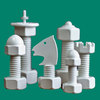
Steven Parker
229,732 PointsTo start with, you have a few syntax issues:
- the if on line 15 needs parentheses around the test expression
- the for on line 29 needs semicolons (;) instead of commas (,)
- the same for is missing an open brace ({)
Fixing those will get it running, but you may want to tweak a few things.
I noticed that even when the correct answer was guessed, the prompts for guesses continue until you use up the entire quota.
Also, getting input with prompt and output to the document seems a bit awkward. You might consider using alert for the responses, or make them part of the next prompt.
To make it challenging, but always doable, limit the guesses to the log(2) of the upper range (rounded up).
Happy coding!

Christopher Borchardt
2,908 Pointsok, i fixed those syntax errors and made a couple of improvements to the code.
it seems to work, except it breaks if i go over 1000 for the guess.
Christopher Borchardt
2,908 PointsChristopher Borchardt
2,908 Pointsok, that really makes it ugly when i copy and pasted the code in, i made a new workspace and took a snapshot.
https://w.trhou.se/3or5ahiaow