Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial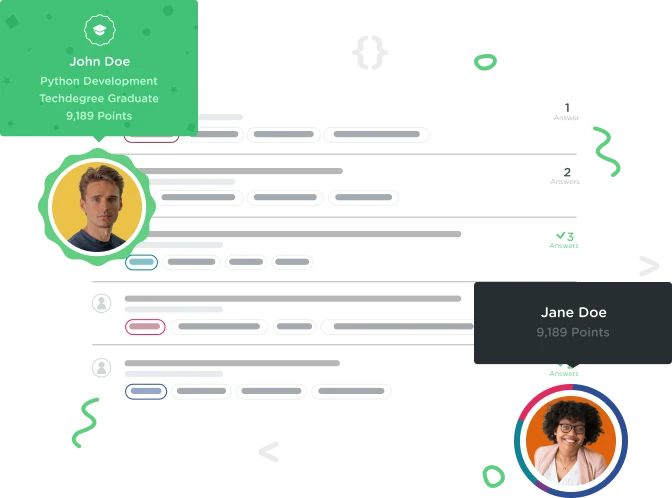
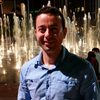
Jeuel Viveros
13,090 PointsGUI not reading input from Binary File. I have three files, the Swing output is correct but no input coming.
import java.awt.*;
import java.io.; import javax.swing.; import java.awt.event.*;
public class EmployeeGUI extends JFrame implements ActionListener
{
private java.util.ArrayList<Employee> empList = new java.util.ArrayList();
private ObjectInputStream input;
private ObjectOutputStream output;
private int noOfEmployee=0;
private int empIndex = 0;
private JTextField TextArea;
private JTextField TextName;
private JTextField TextSalary;
private JPanel Switch;
private JButton UndoBut;
private JButton SaveBut;
private JLabel Message;
private Employee currentEmployee;
private Employee [] employee;
public static final long serialVersionUID = 1L;
public EmployeeGUI () //why does this need to be before the main?
{
setSize(1000, 300);
setTitle("Employee Application");
// addWindowListener(new WindowDestroyer()); DOES IT MATTER WHERE THIS IS
Container content = getContentPane();
content.setBackground(Color.LIGHT_GRAY); //change this from grey to yellow
content.setLayout (new BorderLayout ());
addWindowListener (new WindowDestroyer ());
JPanel aPanel = new JPanel();
aPanel.setBackground(Color.yellow);
aPanel.setLayout(new GridLayout(2,2)); //setting the LAYOUT OF THE PANEL
JPanel aPanel1 = new JPanel ();
aPanel1.add(new JLabel("Employee ID: "));
TextArea = new JTextField (10);
aPanel1.add(TextArea);
aPanel1.setBackground(Color.yellow);
aPanel.add(aPanel1);
aPanel1 = new JPanel();
aPanel1.add(new JLabel ("Employee Name: "));
TextName = new JTextField (20);
aPanel1.add(TextName);
aPanel1.setBackground(Color.yellow);
aPanel.add(aPanel1);
aPanel1 = new JPanel();
aPanel1.add(new JLabel ("Employee Salary: "));
TextSalary = new JTextField (6);
aPanel1.add(TextSalary);
aPanel1.setBackground(Color.yellow);
aPanel.add(aPanel1);
JPanel bPanel2 = new JPanel();
bPanel2.setLayout(new BorderLayout());
JPanel cPanel3 = new JPanel();
cPanel3 = new JPanel();
cPanel3.setBackground(Color.cyan);
Switch = new JPanel();
Switch.setBackground(Color.cyan);
//need to add 4 buttons next, previous , undo and save
JButton aButton = new JButton("Next");
aButton.addActionListener(this);
cPanel3.add(aButton); //adding the button to the panel
aButton = new JButton("Previous");
aButton.addActionListener(this);
cPanel3.add(aButton);
bPanel2.add(cPanel3, "West");
content.add(bPanel2,"South");
UndoBut = new JButton ("Undo");
UndoBut.addActionListener(this);
Switch.add(UndoBut);
UndoBut.setVisible(false);
SaveBut = new JButton ("Save");
SaveBut.addActionListener(this);
Switch.add(SaveBut);
SaveBut.setVisible(false);
bPanel2.add(cPanel3, "West");
bPanel2.add(Switch, "East");
content.add(bPanel2, "South");
content.add (aPanel, "North");
Message = new JLabel();
Message.setForeground(Color.white);
content.add(Message, "Center");
JMenuBar menu = new JMenuBar();
JMenu show = new JMenu("File");
JMenu menu1 = new JMenu ("Modify");
JMenuItem menub = new JMenuItem ("Edit");
menub.addActionListener(this);
menu1.add(menub);
show.add(menu1);
menu1 = new JMenu ("Misc");
menub = new JMenuItem("Exit");
menub.addActionListener(this);
menu1.add(menub);
show.add(menu1);
menu.add(show);
setJMenuBar (menu);
}
public static void main(String[] args)
{
EmployeeGUI gui = new EmployeeGUI();
//gui.initialize(); //DO I NEED THIS
gui.setVisible(true);
}
public void actionPerformed(ActionEvent e)
{
Container content = getContentPane();
String doThis = e.getActionCommand();
if (doThis.equals("Next"))
{
nextEmployee();
}
else if (doThis.equals("Previous"))
{
previousEmployee();
}
else if (doThis.equals("Undo"))
{
undoEdit();
//UndoBut.setVisible(false);
}
else if (doThis.equals("Save"))
{
saveEdit();
//SaveBut.setVisible(false); // see if this works... I need the data to be saved and the button disappeared
}
else if (doThis.equals("Edit"))
{
editData();
UndoBut.setVisible(true);
SaveBut.setVisible(true);
}
else if (doThis.equals("Exit"))
{
Message.setText("Exiting Application after saving data");
saveData();
System.exit(0);
}
else
{
System.out.println("erros in the Comman Interface");
}
}
//now I have to read my data? yes
public void readData()
{
try
{
input = new ObjectInputStream(new FileInputStream("employeedata.dat"));
try
{
noOfEmployee = 0;
while (true)
{
Employee obj;
employee [noOfEmployee]= (Employee) input.readObject();
//empList.add((Employee)input.readObject());
//noOfEmployee +=1;
}
}
catch(EOFException e1)
{
System.out.println("End of File");
}
catch(ClassNotFoundException e2)
{
System.out.println("Class Error:"+ e2.getMessage());
}
System.out.println("Read" +noOfEmployee +"records");
input.close();
}
catch(FileNotFoundException e3)
{
System.out.println("File not found error:" + e3.getMessage());
}
catch(IOException e)
{
System.out.println("Error in the read file" + e.getMessage());
}
}
public void invisible() { TextArea.setEditable(false); TextName.setEditable(false); TextSalary.setEditable(false); UndoBut.setVisible(false); SaveBut.setVisible(false); Switch.setVisible(false);
}
public void initialize ()
{ readData(); loadData(); invisible(); noOfEmployee =empList.size(); empIndex=0; }
public void loadData()
{
currentEmployee =((Employee)empList.get(empIndex));
TextArea.setText(Integer.toString(currentEmployee.getEID()));
TextName.setText(currentEmployee.getEName());
TextSalary.setText(Double.toString(currentEmployee.getESalary()));
}
public void nextEmployee()
{
if (empIndex == noOfEmployee-1)
{
Message.setText("You are at the last record already");
}
else
{
Message.setText("");
empIndex+=1;
loadData();
}
}
public void previousEmployee()
{
if (empIndex==0)
{
Message.setText("You are at the first record already");
}
else
{
Message.setText("");
empIndex-=1;
loadData();
}
}
public void undoEdit()
{
loadData ();
invisible();
}
public void saveEdit ()
{
currentEmployee = ((Employee)empList.get(empIndex));
currentEmployee.setData(Integer.parseInt(TextArea.getText()), TextName.getText(), Double.parseDouble(TextSalary.getText()));
invisible();
}
public void editData()
{
TextArea.setEditable(true);
TextName.setEditable(true);
TextSalary.setEditable(true);
UndoBut.setVisible(true);
SaveBut.setVisible(true);
Switch.setVisible(true);
}
public void saveData()
{
try
{
output = new ObjectOutputStream(new FileOutputStream("employeedata.dat",false));
for(int i=0; i<noOfEmployee;i++)
{
output.writeObject(i);
}
//System.out.println(noOfEmployee+" were written into the file"); DO I NEED IT TO COUND THE FILE?
System.out.println("You Wrote " +noOfEmployee+ " records!");
output.close();
}
catch(IOException e)
{
System.out.println("Error in save data" +e.getMessage());
}
}
} // The employee file is below which is in another class import java.io.Serializable; public class Employee implements Serializable { private int eid; private String ename; private double esalary; public static final long serialVersionUID = 1L;
public Employee(int i, String n, double s)
{
eid = i;
ename = n;
esalary = s;
}
public void setData(int i, String n, double s)
{
eid = i;
ename = n;
esalary = s;
}
public int getEID()
{
return eid;
}
public String getEName()
{
return ename;
}
public double getESalary()
{
return esalary;
}
public String toString()
{
String s = "";
s += "ID = " + eid + " Name = " + ename;
s += " Salary = " + esalary;
return s;
}
}
// the WindowDestroyer file is below
/** *This class is a derived class of the WindowAdapter class. *We only override the windowClosing() method of the *base class. */
//Notice that we import the jawa.awt.event.* package for the WindowAdapter //as well as the WindowEvent class. import java.awt.event.WindowAdapter; import java.awt.event.WindowEvent; public class WindowDestroyer extends WindowAdapter { public void windowClosing(WindowEvent e) { EmployeeGUI en = (EmployeeGUI)e.getSource(); en.saveData(); System.exit(0); } }
// The dat file is called Employee data which I can not upload