Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial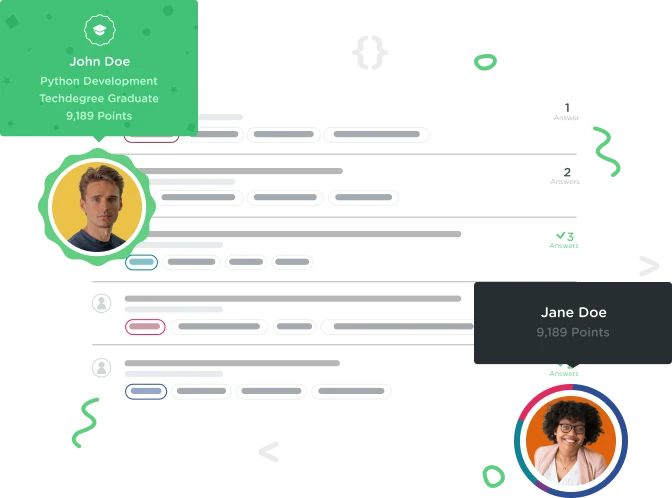

Burhanuddin Oanali
9,939 PointsGuidance
Some days back I had totally given up on this Javascript course as I found it very difficult to follow.
I went and watched the videos more than 5 times and finally its now getting into my head.
I managed to create the to do list appication without referring to the teacher's code. Most of the code looks same..if someone can help to identify if I have made any mistakes in my code..or if I have repetition of code..
// Problem: No user interactivity!
// Solution: Add user interactivity!
var taskInput = document.getElementById("new-task");
var addButton = document.getElementsByTagName("button")[0];
var incompleteTasksHolder = document.getElementById("incomplete-tasks");
var completedTasksHolder = document.getElementById("completed-tasks");
/**********************************
Creating a new list item
**********************************/
var addListItem = function() {
var listItem = document.createElement ("li");
var checkBox = document.createElement("input"); // Type: checkbox
var label = document.createElement("label");
var editInput = document.createElement("input"); // Type: text
var editButton = document.createElement ("button"); // Edit
var deleteButton = document.createElement ("button"); // Delete
/**********************************
Modify Elements
**********************************/
checkBox.type = "checkbox";
editInput.type = "text";
label.innerText = taskInput.value;
editButton.innerText = "Edit Task";
editButton.className = "edit";
deleteButton.innerText = "Delete Task";
deleteButton.className = "delete";
/********************************************
Append the children to its parent (listItem)
********************************************/
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
}
/**********************************
Adding user interactivity
**********************************/
// Add Task
var addTask = function() {
// Print a test message to console.log to check if the function is triggering on clicking add button.
console.log ("Adding new task..");
// Create a new list item..
var listItem = addListItem();
// Append listItem to incomplete-tasks ul
incompleteTasksHolder.appendChild(listItem);
// Clear the task input's value after adding task
taskInput.value = " ";
// Bind the list item to complete-tasks ul when the task is checked.
bindTaskEvents (listItem, completeTasks);
}
// Edit a task
var editTask = function() {
// Print a test message to console.log to check if the function is triggered
console.log("Editing Task");
var listItem = this.parentNode;
var editInput = listItem.querySelector("input[type=text]");
var label = listItem.querySelector ("label");
var editButton = listItem.querySelector("button.edit");
if (listItem.classList.contains("editMode")) {
// Switch from editMode
listItem.classList.toggle("editMode");
// Label text becomes the input's value.
label.innerText = editInput.value;
editButton.innerText = " Edit Task "
}
else {
// Switch to edit mode..
listItem.classList.toggle("editMode");
// Input's value becomes the label text.
editInput.value = label.innerText;
editButton.innerText = " Save Task ";
}
}
// Delete a task
var deleteTask = function() {
// Print a test message to console.log to check if the function is triggered
console.log("Deleting Task");
// When the delete button is pressed..
var listItem = this.parentNode;
var ul = listItem.parentNode;
// Remove the list item from ul..
ul.removeChild(listItem);
}
// Mark task as complete
var completeTasks = function() {
// Print a test message to console.log to check if the function is triggered
console.log("Completing Task");
// When the checkbox is marked as checked..
// Append the task list item to the completed-tasks ul.
var listItem = this.parentNode;
completedTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, incompleteTasks);
}
// Mark task as incomplete
var incompleteTasks = function() {
// Print a test message to console.log to check if the function is triggered
console.log("Incomplete Task");
// When the checkbox is unchecked..
// Append the task list item to the incomplete-tasks ul.
var listItem = this.parentNode;
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, completeTasks);
}
/************************************
Triggering Events
************************************/
addButton.addEventListener("click", addTask);
/******************************************************************************
Bind the list item's children to a particular event (eg editButton to editTask)
******************************************************************************/
var bindTaskEvents = function(taskListItems, checkBoxEventHandler) {
// Select list item's children
var checkBox = taskListItems.querySelector("input[type=checkbox]");
var editButton = taskListItems.querySelector("button.edit");
var deleteButton = taskListItems.querySelector("button.delete");
// Bind the childern to a particular event when triggered.
checkBox.onchange = checkBoxEventHandler;
editButton.onclick = editTask;
deleteButton.onclick = deleteTask
}
// Cycle over the incompleted tasks holder
for (var i = 0; i<incompleteTasksHolder.children.length; i++) {
bindTaskEvents(incompleteTasksHolder.children[i], completeTasks);
}
// Cycle over the completed tasks holder
for (var i = 0; i<completedTasksHolder.children.length; i++) {
bindTaskEvents(completedTasksHolder.children[i], incompleteTasks);
}
2 Answers
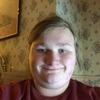
Richard Duffy
16,488 PointsHello,
does your code give you any errors when you run it?
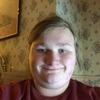
Richard Duffy
16,488 PointsI have put your code in the correct markdown so people can see it more easily :).
// Problem: No user interactivity!
// Solution: Add user interactivity!
var taskInput = document.getElementById("new-task");
var addButton = document.getElementsByTagName("button")[0];
var incompleteTasksHolder = document.getElementById("incomplete-tasks");
var completedTasksHolder = document.getElementById("completed-tasks");
/**********************************
Creating a new list item
**********************************/
var addListItem = function() {
var listItem = document.createElement ("li");
var checkBox = document.createElement("input"); // Type: checkbox
var label = document.createElement("label");
var editInput = document.createElement("input"); // Type: text
var editButton = document.createElement ("button"); // Edit
var deleteButton = document.createElement ("button"); // Delete
/**********************************
Modify Elements
**********************************/
checkBox.type = "checkbox";
editInput.type = "text";
label.innerText = taskInput.value;
editButton.innerText = "Edit Task";
editButton.className = "edit";
deleteButton.innerText = "Delete Task";
deleteButton.className = "delete";
/********************************************
Append the children to its parent (listItem)
********************************************/
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
}
/**********************************
Adding user interactivity
**********************************/
// Add Task
var addTask = function() {
// Print a test message to console.log to check if the function is triggering on clicking add button.
console.log ("Adding new task..");
// Create a new list item..
var listItem = addListItem();
// Append listItem to incomplete-tasks ul
incompleteTasksHolder.appendChild(listItem);
// Clear the task input's value after adding task
taskInput.value = " ";
// Bind the list item to complete-tasks ul when the task is checked.
bindTaskEvents (listItem, completeTasks);
}
// Edit a task
var editTask = function() {
// Print a test message to console.log to check if the function is triggered
console.log("Editing Task");
var listItem = this.parentNode;
var editInput = listItem.querySelector("input[type=text]");
var label = listItem.querySelector ("label");
var editButton = listItem.querySelector("button.edit");
if (listItem.classList.contains("editMode")) {
// Switch from editMode
listItem.classList.toggle("editMode");
// Label text becomes the input's value.
label.innerText = editInput.value;
editButton.innerText = " Edit Task "
}
else {
// Switch to edit mode..
listItem.classList.toggle("editMode");
// Input's value becomes the label text.
editInput.value = label.innerText;
editButton.innerText = " Save Task ";
}
}
// Delete a task
var deleteTask = function() {
// Print a test message to console.log to check if the function is triggered
console.log("Deleting Task");
// When the delete button is pressed..
var listItem = this.parentNode;
var ul = listItem.parentNode;
// Remove the list item from ul..
ul.removeChild(listItem);
}
// Mark task as complete
var completeTasks = function() {
// Print a test message to console.log to check if the function is triggered
console.log("Completing Task");
// When the checkbox is marked as checked..
// Append the task list item to the completed-tasks ul.
var listItem = this.parentNode;
completedTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, incompleteTasks);
}
// Mark task as incomplete
var incompleteTasks = function() {
// Print a test message to console.log to check if the function is triggered
console.log("Incomplete Task");
// When the checkbox is unchecked..
// Append the task list item to the incomplete-tasks ul.
var listItem = this.parentNode;
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, completeTasks);
}
/************************************
Triggering Events
************************************/
addButton.addEventListener("click", addTask);
/******************************************************************************
Bind the list item's children to a particular event (eg editButton to editTask)
******************************************************************************/
var bindTaskEvents = function(taskListItems, checkBoxEventHandler) {
// Select list item's children
var checkBox = taskListItems.querySelector("input[type=checkbox]");
var editButton = taskListItems.querySelector("button.edit");
var deleteButton = taskListItems.querySelector("button.delete");
// Bind the childern to a particular event when triggered.
checkBox.onchange = checkBoxEventHandler;
editButton.onclick = editTask;
deleteButton.onclick = deleteTask
}
// Cycle over the incompleted tasks holder
for (var i = 0; i<incompleteTasksHolder.children.length; i++) {
bindTaskEvents(incompleteTasksHolder.children[i], completeTasks);
}
// Cycle over the completed tasks holder
for (var i = 0; i<completedTasksHolder.children.length; i++) {
bindTaskEvents(completedTasksHolder.children[i], incompleteTasks);
}