Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial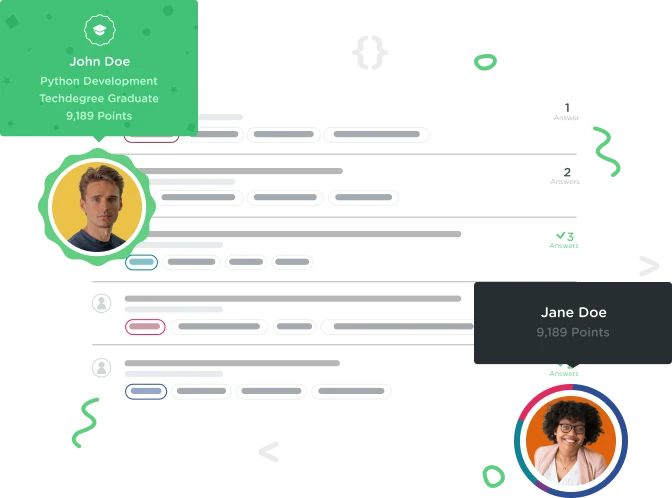

Donna Lorenzo
Courses Plus Student 7,150 PointsGuil's Up and Down Button Challenge
Hello Treehouse Community,
I need your help explaining what is happening in a section of my code. I think I solved Guil's challenge by mistake. I created a function that hides the buttons and called the function in the event listener. What i don't understand is why do the buttons stay in place if I call the function inside event and if I call the function outside they move. Thank you very much in advance!
// Hides unused buttons
function hideUnusedButtons() {
let firstListItem = listUl.firstElementChild;
let lastListItem = listUl.lastElementChild;
firstListItem.firstElementChild.style.visibility = 'hidden';
lastListItem.querySelector('.down').style.visibility = 'hidden';
for (let i = 0; i < lis.length; i++) {
if(lis[i] !== firstListItem) {
lis[i].firstElementChild.style.visibility = 'visible';
}
if(lis[i] !== lastListItem) {
lis[i].querySelector('.down').style.visibility = 'visible';
}
}
}
// Moves items up and down and removes them
listUl.addEventListener('click', (event) => {
if (event.target.tagName == 'BUTTON') {
if (event.target.className == 'remove') {
let li = event.target.parentNode;
let ul = li.parentNode;
ul.removeChild(li);
}
if (event.target.className == 'up') {
let li = event.target.parentNode;
let prevLi = li.previousElementSibling;
let ul = li.parentNode;
if (prevLi !== null) { // Checking to see if Li has a previous element
ul.insertBefore(li, prevLi);
}
}
if (event.target.className == 'down') {
let li = event.target.parentNode;
let nextLi = li.nextElementSibling;
let ul = li.parentNode;
if (nextLi !== null) { // Checking to see if Li has an after element
ul.insertBefore(nextLi, li);
}
}
}
hideUnusedButtons();
});