Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial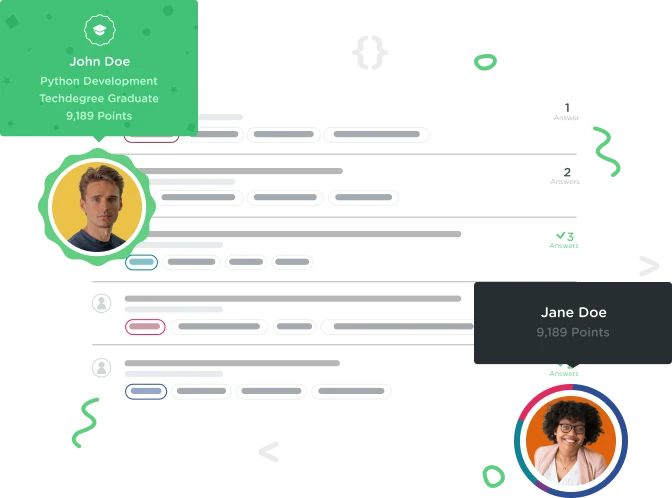

John Perry
Full Stack JavaScript Techdegree Graduate 41,012 PointsGulp Basics: How to update from Gulp 3 to Gulp 4
So I went through the "Gulp Basics" course and I ran into a bunch of errors when trying to compile the final project. It took some adjustments of the package.json file so I wanted to outline the steps.
1. Change all tasks that have 3 parameters
If you're getting this error:
AssertionError [ERR_ASSERTION]: Task function must be specified
the following will fix the issue. Find any task that looks like this:
gulp.task("taskName", ["otherTasks"], function () {
/* other code */
}))
and change it to this:
gulp.task("taskName", gulp.series(["otherTasks"], function () {
/* other code */
}))
Don't forget to add a closing parenthesis since there's a new one after gulp.series(
2. Install the gulp-cli globally
If you're now getting this error:
TypeError: Cannot read property 'apply' of undefined
the following will fix the issue. In the cli (e.g. terminal or CMD), type this:
npm i -g gulp-cli
3. Update the syntax for the watchFiles task
Assuming you've tried compiling your code after each step, the next (and final) error you'll run into is caused by the syntax in the watchFiles
task.
Update this:
gulp.watch("scss/**/*.scss", ["compileSass"])
gulp.watch("js/main.js", ["concatScripts"])
to this:
gulp.watch("scss/**/*.scss", gulp.parallel(["compileSass"]))
gulp.watch("js/main.js", gulp.parallel(["concatScripts"]))
At this point, if the code is not compiling, throw out your computer and buy a new one.