Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial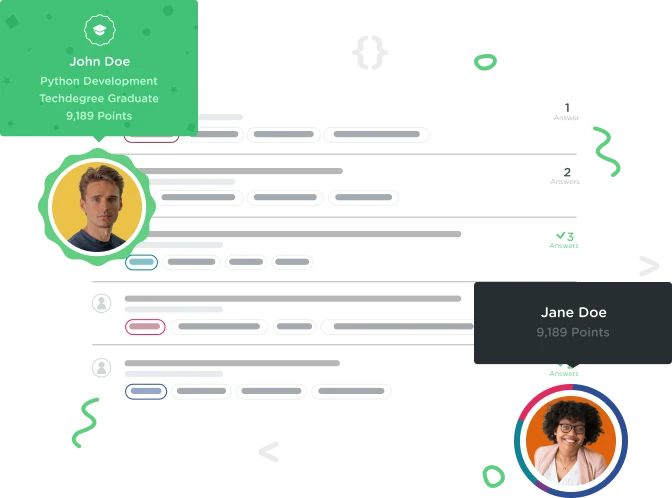
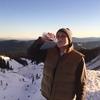
Nick Casey
1,840 PointsHa, so I may be a little off here....
I'm trying to structure the following .js file as a loop that allows a user to input the answer "sesame" before receiving a successful return prompt.
var secret = prompt("What is the secret password?");
varCount +=1;
if (parseInt(guess) = "sesame") {
correctGuess = true;
}
while (! correctGuess )
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="app.js"></script>
</body>
</html>
3 Answers

Brent Suggs
Front End Web Development Techdegree Graduate 21,343 PointsThere are a couple of issues here. First off in your 'if' statement you are not using a comparison but rather trying to assign a value. Singe '=' is always goign to assign a value so you should be using either '==' or '===' depending on how explict of a match you are looking for.
var secret = prompt("What is the secret password?");
varCount +=1;
if (parseInt(guess) == "sesame") {
correctGuess = true;
}
while (! correctGuess )
document.write("You know the secret password. Welcome.");
Also, where are you defining the variable 'correctGuess'?
It looks as thought you are defining it on the fly inside of your if statement. It should be declared and set to false as a default, but in all honestly you don't need that variable at all.
Instead you can just use if .. else:
var secret = prompt("What is the secret password?");
varCount +=1;
if (parseInt(guess) == "sesame") {
document.write("You know the secret password. Welcome.");
} else {
document.write("You do not know the password.");
}
Or you could prompt for an answer again in the else statement.
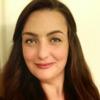
Jennifer Nordell
Treehouse TeacherOk, before I start pointing out errors, I'd like to say that you're doing great! First, you have something called varCount which is a variable that was never declared, but then you try to use. That's going to create an error. Secondly a while loop starts with the while a condition is true. It seems like you were trying to write the while at the bottom sort of like we'd see in a do while loop (which is slightly different). Third, we don't want to try to parse an integer out of the person's guess. We want it to be "sesame"... not a number. Take a look at my solution and see if you can see what I mean:
var secret = prompt("What is the secret password?");
while(secret !== "sesame") {
secret = prompt("Try again!");
}
document.write("You know the secret password. Welcome.");

Brent Suggs
Front End Web Development Techdegree Graduate 21,343 PointsAlso, a good way to go... :)
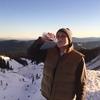
Nick Casey
1,840 PointsAlright, Jennifer and Brent, thank you for both of your great answers. Although Jennifer's solution used the least amount of code, I was able to intuitively digest mr. Brandon's else-if example much easier. I got a little tripped up when keeping up with the !== not equal to. Does this almost approach the loop from the perspective of a double-negative? .....(obviously such a noob over here) super interesting stuff, though. Thanks x1000000 :-)