Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial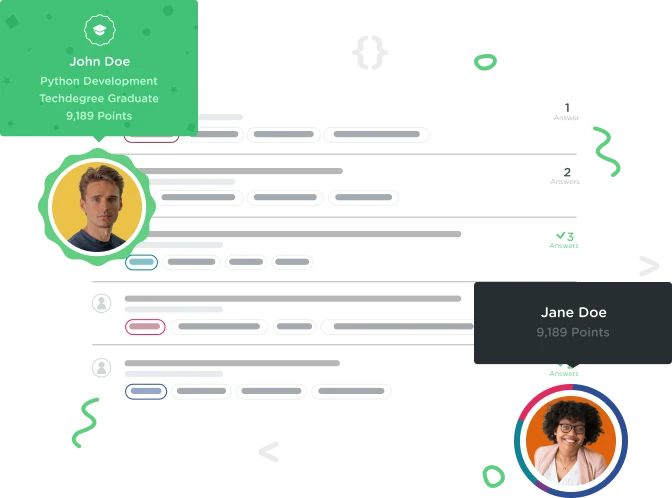
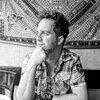
jlampstack
23,932 PointsHack to fix broken API...
Here's a hack to help fix the broken API. I used this hack recommended by another Treehouse member in the earlier stages of the project.
** If any moderators can approve this post verify it's okay, it would be very helpful, as I'm still learning this topic and don't want to offer wrong information **
const astrosUrl = 'http://api.open-notify.org/astros.json';
const wikiUrl = 'https://en.wikipedia.org/api/rest_v1/page/summary/';
const peopleList = document.getElementById('people');
const btn = document.querySelector('button');
// Handle all fetch requests
async function getPeopleInSpace(url) {
const peopleResponse = await fetch(url);
const peopleJSON = await peopleResponse.json();
const profiles = peopleJSON.people.map( async (person) => {
if (person.name === "Anatoly Ivanishin") {
person.name = "Anatoli Ivanishin";
}
if (person.name === "Chris Cassidy") {
person.name = "Christopher Cassidy";
}
const craft = person.craft;
const profileResponse = await fetch(wikiUrl + person.name);
const profileJSON = await profileResponse.json();
return { ...profileJSON, craft };
});
return Promise.all(profiles);
}
// Generate the markup for each profile
function generateHTML(data) {
data.map( person => {
const section = document.createElement('section');
peopleList.appendChild(section);
section.innerHTML = `
<img src=${person.thumbnail.source}>
<span>${person.craft}</span>
<h2>${person.title}</h2>
<p>${person.description}</p>
<p>${person.extract}</p>
`;
});
}
btn.addEventListener('click', async (event) => {
event.target.textContent = 'Loading...';
const astros = await getPeopleInSpace(astrosUrl);
generateHTML(astros);
event.target.remove();
});
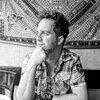
jlampstack
23,932 PointsYes, this one by Viktor Lovgren

Martin Coton
3,015 PointsI don't think anyone cares that this series of videos is dense and largely impenetrable to new coders. "Read the teachers notes below" - great, I read them and am taken to a similar, sparsely detailed article (usually on MSDN) which leaves me wondering why I'm paying to be "taught" the subject in the first place. There are so many free explanantions of almost every aspect of JavaScript out there.
The fix shown above is an appalling bodge-up. Students should NOT be encouraged to use this example just to get through the tutorial. Treehouse need to address the fact that a 404 is breaking the project during these lessons. The generateHTML function is passed an undefined item in the object array, breaking the app when data.map tries to read the value person.thumbnail.source.
Wrapping the html bulder in a conditional stops the app breaking, but then there's the unhandled 404 sitting in the console. We need to know how to deal with situations like that too.
function generateHTML(data) {
data.map( person => {
if(person.title !== "Not found."){
const section = document.createElement('section');
peopleList.appendChild(section);
section.innerHTML = `
<img src=${person.thumbnail.source}>
<span>${person.craft}</span>
<h2>${person.title}</h2>
<p>${person.description}</p>
<p>${person.extract}</p>
`;
}
});
}
3 Answers
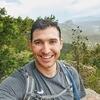
Justin Hein
14,811 PointsI agree that this is not a great look for Treehouse. An API should be used which returns more standardized data.
Still, I think this is a solution that is fairly reliable, even more so than checking the .title
on a person object.
function generateHTML(data) {
data.map( person => {
if (person.type == "standard") {
const section = document.createElement('section');
peopleList.appendChild(section);
section.innerHTML = `
<img src=${person.thumbnail.source}>
<span>${person.craft}</span>
<h2>${person.title}</h2>
<p>${person.description}</p>
<p>${person.extract}</p>
`;
}
});
}
This ensures that the right .type
of page has been found. It could also be a fun challenge to iterate on this. Maybe create an additional function that generates an option for the user to choose which profile from Wikipedia they're looking for and load it once they've chosen?
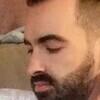
Jesse Cleary-Budge
Front End Web Development Techdegree Graduate 15,359 PointsI also agree this exercise creates a lot of opportunities for it to break on its own as astronauts with more or less ambiguous names leave and enter space. It would be awesome to see Treehouse challenge us to account for this and to handle the attendant errors.
I took a similar approach to Justin Hein above by analyzing the JSON from Wikipedia and filtering to present the user with some relevant information (of course this could be improved upon even more as suggested above):
function generateHTML(data) {
data.map( person => {
const section = document.createElement('section');
peopleList.appendChild(section);
if ( person.type === 'standard' ) {
section.innerHTML = `
<img src=${person.thumbnail.source}>
<h2>${person.title}</h2>
<p>${person.description}</p>
<p>${person.extract}</p>
`;
} else {
section.innerHTML = `
<img src='http://via.placeholder.com/200' alt='placeholder'>
<h2>${person.title}</h2>
<p>${person.extract_html}</p>
`;
}
});
}

wc93
25,725 PointsTreehouse needs to step it up. I have been able to make it through everything fairly easily up to this point. There is much outdated content and it does not seem they are actively fixing issues or updating quickly enough. I just about gave up on this when my fixes were thrown to the wind with the Promise.all(). Seems like many of these courses start out well but then the last half is sped through with little instruction of real value. Going through the code in dev tools line by line helps me greatly. Thank you to those who created solutions but being able to parse and handle exceptions and/or errors is what needs greater focus in my opinion. Thank you to Jesse Cleary-Budge for his solution that at least shows you Who is in Space. I kept thinking about the Muppet's Pigs in Space throughout this course. Onward and Upward.
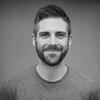
Daniel Cranney
11,609 PointsI completely agree wc93 , it's not a good look for them. Up until now I've been really happy with the treehouse content, but this is the most complicated aspect of the frontend development course by far, and all I get is a blank screen when you click the button. Considering the monthly fee, and the amount of people using their resources, it should go without saying that quality, ACCURATE content is what we pay for. This really is enough for me to consider going elsewhere (codecademy etc) because Treehouse just don't seem to care.
Steven Parker
231,275 PointsSteven Parker
231,275 PointsI assume you're referring to the code suggestion made by Viktor Lovgren in this previous question.