Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial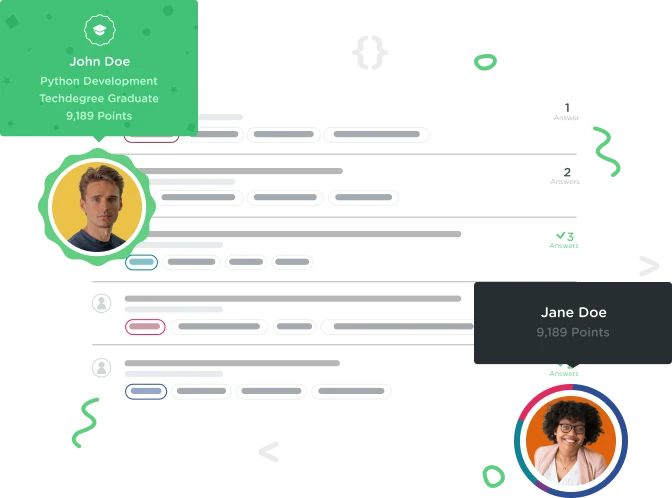

Bart Christoffersen
759 PointsHad an idea and can't get it to work
I had a hairbrain idea to try to make my own little program but I can't get it to work and wondered if I could get some help trying to apply some of the principles I have picked up.
All I get in the console is the number 0.
//I want to roll a die and see the sum of all the times it takes to get a 0, which will be a jackpot and alert the user that it took them X number of times to get the jackpack. I figure that landing directly on the number 0 in Math.random are slim. So, I wanted to make a game of it.
//create the array that will store the history of die rolls
var rollHistory = [];
//create variable to hold the total die rolls counter
var totalDieRolls = 0;
//create variable to hold the die roll parameter
var dieRoll = 1;
//loop function until you roll a 0, then display the number of times it looped to get to roll zero
var loop = function(){
while(dieRoll > 0) {
//generate a random number
var randomNumber = Math.random();
console.log("Random number = " + randomNumber);
//return an integer between 0 and 6
var dieRoll = Math.ceil(randomNumber * 6);
console.log("Random die roll = " + dieRoll);
rollHistory.push(dieRoll);
console.log(rollHistory);
totalDieRolls++;
console.log(totalDieRolls);
}
}
loop();
console.log(totalDieRolls);
alert("It took " + totalDieRolls + "to land the jackpot!!!")
I was trying to debug it by keeping an eye on the console (thus all the console.log()). But I get only the one response.
2 Answers

Rand Seay
13,975 PointsI think you are scoping your dieRoll
variable incorrectly. If you replace var dieRoll
with simply dieRoll
inside your loop, then the code runs and the console goes crazy (which I think you are intending).
//return an integer between 0 and 6
//var dieRoll = Math.ceil(randomNumber * 6);
dieRoll = Math.ceil(randomNumber * 6);
console.log("Random die roll = " + dieRoll);
Just so you know, this isn't far from an endless loop, and can freeze up your browser (Source: I did it).
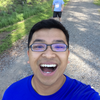
Jarold Wong
7,766 PointsHi there, I think first thing you need to do is move the variable declarations outside the loop.
var loop = function(){
var randomNumber = Math.random();
var dieRoll = Math.ceil(randomNumber * 6);
while(dieRoll > 0) {
//generate a random number
randomNumber = Math.random();
console.log("Random number = " + randomNumber);
//return an integer between 0 and 6
dieRoll = Math.ceil(randomNumber * 6);
Bart Christoffersen
759 PointsBart Christoffersen
759 PointsYa, I had to kill it a lot. I wonder if the odds are just so low to get Math.random() to return 0, cause when i fixed it, it still loops. But when it does go, I don't get anything in the log telling me what it was doing. Maybe it was an effort in futility, but thought it would be a fun exercise. Thanks for the help.