Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial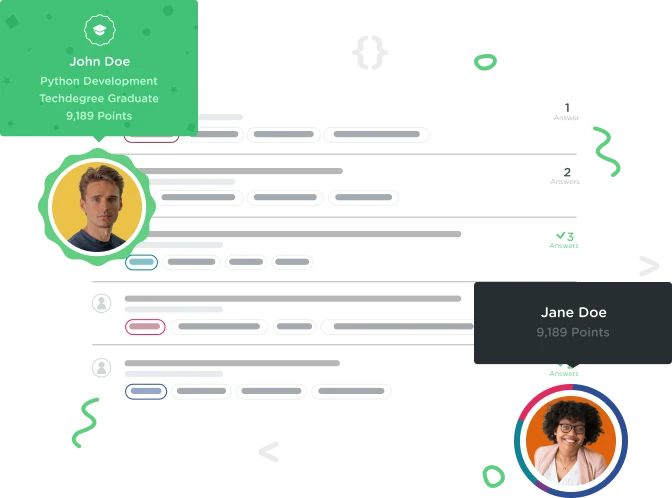

Daniel Meles
1,459 Pointshalf my code won't run
Here is my code
class Example {
public static void main(String[] args) {
System.out.println("we are makeing a a new pez disenser");
PezDispenser dispenser = new PezDispenser("Yoda");
System.out.printf("Fun fact a pez dispenser can only hold %d pez %n",
dispenser.MAX_PEZ);
System.out.printf("The Dispenser is %s %n",
dispenser.getCharecterName());
if(dispenser.isEmpty()) {
System.out.println("Your Dispenser is empty");
System.out.println("________________________________");
System.out.println("Filling your PezDispenser ... ");
dispenser.fill();
}
if(dispenser.getPezCount() == dispenser.MAX_PEZ) {
System.out.println("________________________________");
System.out.println("Your Pez Dispenser is full");
System.out.println("________________________________");
} else {
System.out.println("________________________________");
System.out.printf("You have %d pez %n",
dispenser.getPezCount());
System.out.println("________________________________");
}
while(dispenser.getPezCount() > 0){
System.out.println("CHOMP! ...");
dispenser.eatOne();
}
if(dispenser.isEmpty()){
System.out.println("You ate all the pez");
}
dispenser.fill(2);
dispenser.fill(6);
System.out.println("Added 8 pez");
while(dispenser.getPezCount() > 0){
System.out.println("CHOMP! ...");
dispenser.eatOne();
}
try {
dispenser.fill(100);
System.out.println("Adding 100 Pez");
} catch(IllegalArgumentException iae) {
System.out.printf("Error %nError : %s %n",iae.getMessage());
}
}
}
and here is the output
Picked up JAVA_TOOL_OPTIONS: -Xmx128m
Picked up _JAVA_OPTIONS: -Xmx128m
Picked up JAVA_TOOL_OPTIONS: -Xmx128m
Picked up _JAVA_OPTIONS: -Xmx128m
we are makeing a a new pez disenser
Fun fact a pez dispenser can only hold 12 pez
The Dispenser is Yoda
Your Dispenser is empty
________________________________
Filling your PezDispenser ...
________________________________
You have 0 pez
________________________________
You ate all the pez
Added 8 pez
Error
Error : Too many Pez
if you need it here is my PezDispenser.java
class PezDispenser {
public static final int MAX_PEZ = 12;
private String charecterName;
private int pezCount;
public PezDispenser(String charecterName) {
this.charecterName = charecterName;
}
public boolean isEmpty() {
return pezCount == 0;
} //isEmpty()
public void fill() {
fill(MAX_PEZ);
} //fill()
public void fill(int pezAmount) {
int newAmount = pezCount + pezAmount;
if(newAmount > MAX_PEZ) {
throw new IllegalArgumentException("Too many Pez");
}
newAmount = pezCount;
}
public void addOne() {
pezCount ++;
} //addOne()
public void eatOne() {
pezCount--;
} //eatOne()
public String getCharecterName() {
return charecterName;
} //getCharecterName()
public int getPezCount() {
return pezCount;
} //getPezCount()
}
1 Answer

andren
28,558 PointsThe fill(int pezAmount) method does not actually fill the PezDispenser because the last line:
newAmount = pezCount;
Is the opposite of what it should be, you are meant to set the pezCount equal to the newAmount like this:
pezCount = newAmount;
Otherwise you will always end up with 0 pez in the dispenser.
Fixing that error should make your code act as intended.