Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial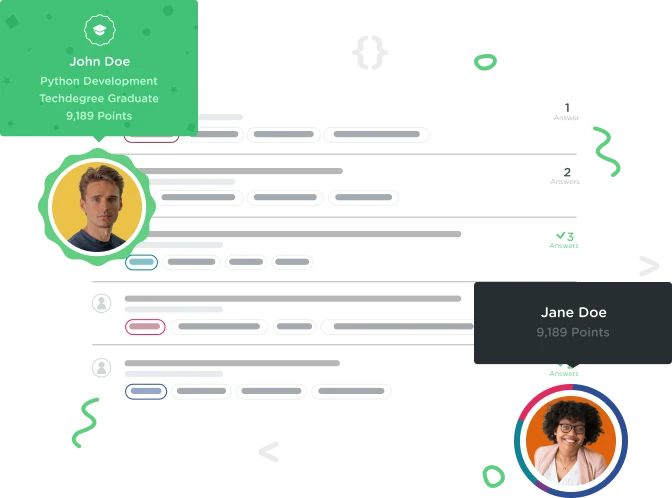

Scott Baumbich
Courses Plus Student 22,129 PointsHandling Errors Coding Challenge
Hello Community,
I'm hung up on this problem and need some assistance. A better explanation of the question with the use of an example would be helpful. Q&A below:
Q) Now that you have good error handling code, let's call the parse method. Remember that since this is a throwing function, you will need to use a do catch block. For this task, just use a generic catch block rather than pattern matching on specific errors. In the catch clause, simply log an error message of your choosing.
enum ParserError: ErrorType {
case EmptyDictionary
case InvalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
guard let someData = data else {
throw ParserError.EmptyDictionary
}
guard let someKey = data!["someKey"] else {
throw ParserError.InvalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)
6 Answers
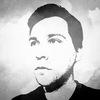
dmnk
18,695 PointsHey, this works:
enum ParserError: ErrorType {
case EmptyDictionary
case InvalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
guard let _ = data else {
throw ParserError.EmptyDictionary
}
guard let _ = data!["someKey"] else {
throw ParserError.InvalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil]
do {
let parser = try Parser(data: data).parse()
} catch {
print("Error")
}

jakesager
12,023 PointsI know I'm late, but here's what I came up with that works. It doesn't require force unwrapping using the bang operator.
func parse() throws {
// unwrapping data into someData, be sure to use someData from now on
guard let someData = data else {
throw ParserError.EmptyDictionary
}
// if statement to check whether it has the key
if !someData.keys.contains("someKey") {
throw ParserError.InvalidKey
}
}
}

vicentelee
6,662 Pointsthis should be best answer

John Hendrix
21,844 PointsI had capitol letter errors in the above code. This worked for me:
enum ParserError: Error {
case emptyDictionary
case invalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
// unwrapping data into someData, be sure to use someData from now on
guard let someData = data else {
throw ParserError.emptyDictionary
}
// if statement to check whether it has the key
if !someData.keys.contains("someKey") {
throw ParserError.invalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil]
do {
let parser = try Parser(data: data).parse()
} catch {
print("Error")
}

Heather Mathewson
10,912 PointsI am also having trouble with this. Did you figure it out?

Scott Baumbich
Courses Plus Student 22,129 PointsNot yet, I've just skipped ahead. If anything changes I'll let you know.

Scott Baumbich
Courses Plus Student 22,129 PointsNot yet, I've just skipped ahead. If anything changes I'll let you know.
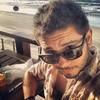
james rochabrun
Courses Plus Student 22,726 Pointsenum ParserError: Error { case emptyDictionary case invalidKey }
struct Parser { var data: [String : String?]?
func parse() throws {
guard (data?["someKey"]) != nil else {
throw ParserError.invalidKey
}
guard (data?.keys) != nil else {
throw ParserError.emptyDictionary
}
}
}
let data: [String : String?]? = ["someKey": nil] let parser = Parser(data: data)
do { try parser.parse() } catch ParserError.invalidKey { print(ParserError.invalidKey) }

Carl Grape
Python Web Development Techdegree Student 10,133 PointsSuper simple:
do { try parser.parse() } catch { print("nope") }
Heather Mathewson
10,912 PointsHeather Mathewson
10,912 PointsThat worked! Can you explain the use of the _ after both let's up above? While xcode recommended it to me, I wasnt sure why.
dmnk
18,695 Pointsdmnk
18,695 PointsXcode recommended it because both parameters will not be used in the following statement. _ is a placeholder parameter name. It indicates that a parameter is expected, but will not be used.