Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial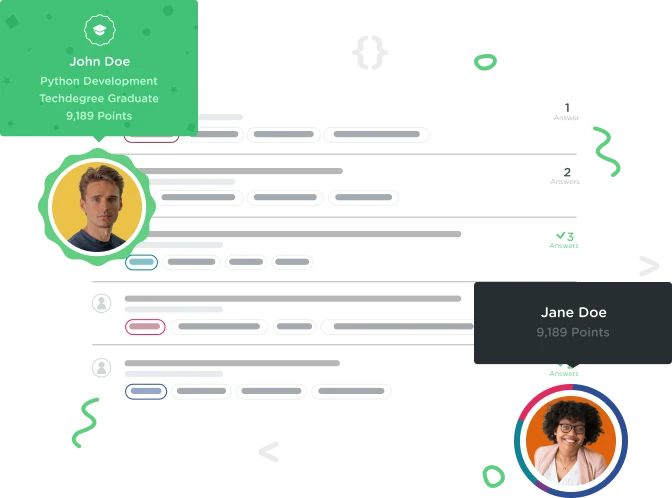

Feng Chen
2,488 PointsHandling Exception: Is the first line is System.out.println necessary?
Ok, so this is the correct answer. However, I tried this code without the line 11: System.out.println("Error!"); and it would be incorrect answer. What I want to know is if this line is necessary, isn't line 12 doing the similar job?
Thanks.
public class Main {
public static void main(String[] args) {
GoKart kart = new GoKart("yellow");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
try{
kart.drive(2);
}
catch (IllegalArgumentException e){
System.out.println("Error!");
System.out.printf("Error! %s" , e.getMessage());
}
}
}
2 Answers

Derek Markman
16,291 PointsTo simply answer your question, no, since both of your print statements are doing similar operations. It's a bit redundant to add that first println statement. The only difference between your print statements is that your printf statement is invoking the 'getMessage()' method.
By looking at the Throwable class documention, it shows that the 'getMessage()' method returns the detail message string of this throwable.
"Returns: the detail message string of this Throwable instance (which may be null)." - from the documentation.
You can invoke the getMessage method from a println or printf statement like so:
//Exception variable name of 'e'
System.out.println("Something went wrong " + e.getMessage()); //println statement
System.out.printf("Something went wrong %s", e.getMessage()); //printf statement
Here's a basic example of the getMessage() method just so you have a better idea of how it works:
import java.io.*;
public class ExceptionPractice {
public static void main(String[] args) {
try {
methodThatThrowsAnIOException();
} catch (IOException ioe) {
System.out.println("Yikes!: " + ioe.getMessage());
}
}
private static void methodThatThrowsAnIOException() throws IOException {
throw new IOException("An IOException occurred");
}
}
The output for the above example is:
Yikes!: An IOException occurred
Regarding the code challenge that's testing you on exception handling, in the first code challenge 'throwing exceptions'. It say's (Okay, so let's throw an IllegalArgumentException from the drive method if the requested number of laps cannot be completed on the current battery level. Make the exception message "Not enough battery remains").
So, if mBarsCount is less than the number of laps, an IllegalArgumentException is thrown from your drive method, and your getMessage will log "Not enough battery remains". Said IllegalArgumentException is caught and handled in your try-catch block that you added your print statement to in the second code challenge "Handling exceptions".
In your catch block, if you add something like System.out.println("Uh-oh: " + e.getMessage()); your output would be: "Uh-oh: Not enough battery remains".
Let me know if you have any other questions, happy coding. :)
If you want to read more about the getMessage method/Throwable class you can find it here: https://docs.oracle.com/javase/7/docs/api/java/lang/Throwable.html#getMessage()
And you can also read more about the Exception class here: https://docs.oracle.com/javase/7/docs/api/java/lang/Exception.html

Feng Chen
2,488 PointsThanks for your help! :D

Derek Markman
16,291 PointsNo problem Feng, let me know if you have any other questions.
Feng Chen
2,488 PointsFeng Chen
2,488 PointsObviously we can't see the line with attachment file, so line 12 is referring to "System.out.printf("Error! %s", e.getMessage());