Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial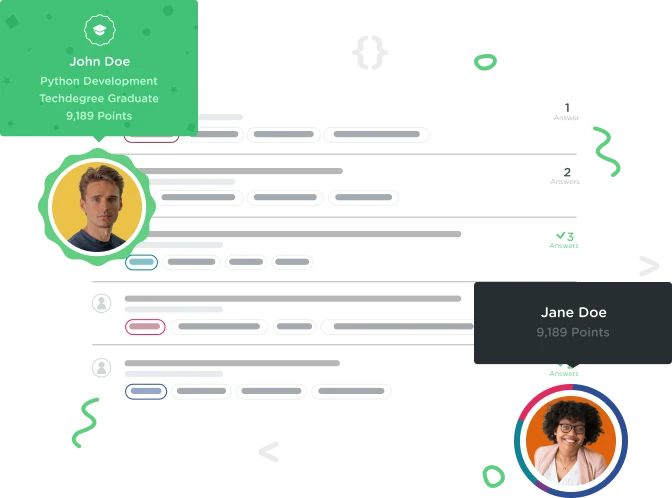

Mohamed Tawfik
Courses Plus Student 19,338 PointsHandling exceptions in JUnit 5
I can't handle exceptions in JUnit 5 Neither by the Expected keyword nor by the Rule annotation
4 Answers

Miguel Nunez
1,081 Points@Test
void overStockingNotAllowed() {
assertThrows(IllegalArgumentException.class, () ->
bin.restock("Mars", 15, 100, 50),
"There are only 10 spots left");

Daniel Marin
8,021 PointsI'm using junit5 as well here's an example for the vending project:
@Test
void notEnoughFundsToDeduct() {
Assertions.assertThrows(NotEnoughFundsException.class, () -> creditor.deduct(10));
}
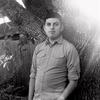
Oziel Perez
61,321 PointsPer the docs:
@Test
void exceptionTesting() {
Throwable exception = assertThrows(IllegalArgumentException.class, () -> {
throw new IllegalArgumentException("a message");
});
assertEquals("a message", exception.getMessage());
}
@Rule no longer exists in JUnit 5 and is superseded by @ExtendedWith. However, there is a migration guide which helps continue to use the @Rule annotation, but this is recommended only to those that have large fixtures with multiple rules and don't wish to rewrite all their tests right away.

Muhammad Nagy
944 PointsIn JUnit 5, the test should be rewritten like the following
@Test
void overstockingNotAllowed() {
IllegalArgumentException thrown =
assertThrows(
IllegalArgumentException.class,
() -> {
bin.restock("Fritos", 2600, 100, 50);
});
assertTrue(thrown.getMessage().equals("There are only 10 spots left"));
}
Kjetil Lorentzen
13,360 PointsKjetil Lorentzen
13,360 PointsHi Mohamed,
I got into the same problem you were experiencing after watching the videos and using JUnit5. After some googling I found that there is a new (and perhaps improved) way of doing unit testing when testing for expected messages from thrown exceptions.
See below for my implementation: