Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial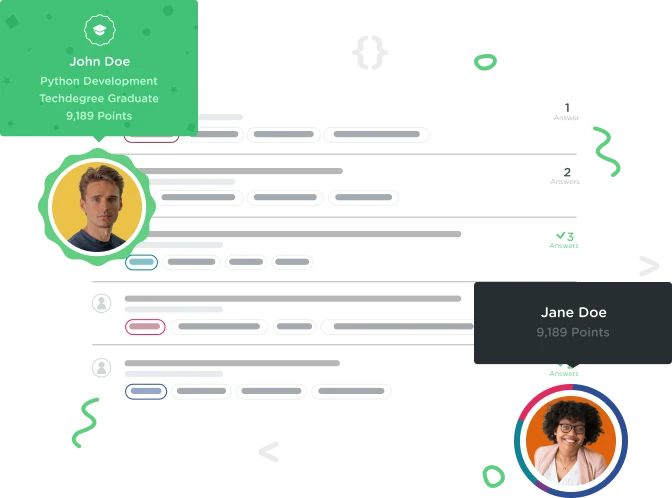
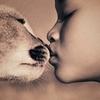
melissakeith
3,766 PointsHandling exceptions task
I'm not sure what i'm doing wrong in this code.
public class Main {
public static void main(String[] args) {
GoKart kart = new GoKart("yellow");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
kart.drive(2);
try {
kart.drive(3);
System.out.println("Only one lap per bar");
} catch (IllegalArgumentException iae) {
System.out.println("Limit exceeded!");
System.out.printf("The error was: %s\n", iae.getMessage());
}
}
}
1 Answer

Andrew Winkler
37,739 PointsThere's a lot going on here & in this exercise in general. here is part 2 of the code challenge. You may have labeled your exceptions differently, but even so I anticipate you are "try"ing too much ;D
If you want to read up, here's the oracle documentation.
public class Main {
public static void main(String[] args) {
GoKart kart = new GoKart("yellow");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
// wrap potentially dangerous code in the try block
try {
kart.drive(2);
}
// catch an illegal argument exception e and use e to get the message
catch (IllegalArgumentException e) {
System.out.println(e.getMessage());
}
}
}