Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial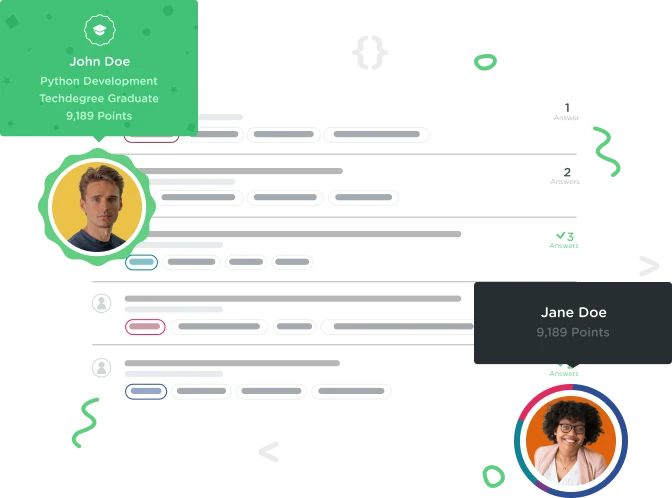
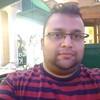
Ashwin Kumar
680 PointsHandling multiple ValueError exceptions
Hi, I loved the idea of managing user errors and it has opened up new thinking for me. So I experimented with the split check script a little more by adding an exception to the total amount if it is less than 1. I'm pasting the code here. I'm not sure what's wrong, but the second print argument"Please enter a valid number" is not getting printed. Please help where I'm going wrong.
import math def split_check(total, number_of_people): if number_of_people <= 1: raise ValueError("More than one person is required to split the check") cost_per_person = math.ceil(total / number_of_people) if total <= 1: raise ValueError("Need a valid positive amount to continue") return cost_per_person try: total_amount = float(input("Enter the total amount: ")) total_people = int(input("Enter the total number of people: ")) amount_due = split_check(total_amount, total_people)
except ValueError as err2: print("({})".format(err2))
except ValueError as err: print("({})".format(err)) print("Please enter a valid number")
else: print("Total amount to be paid by each person is:", amount_due)
Please tell me what I'm doing wrong.
Also, sorry I don't know how to Markdown this!
2 Answers
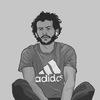
youssef b10ta
Courses Plus Student 2,755 Pointsimport math
def split_check(total, number_of_people):
if number_of_people <= 1 or total <= 1:
raise ValueError("More than one person is required to split the check")
if total <= 1:
raise ValueError("Please enter an amount larger than 1")
return math.ceil(total / number_of_people)
try:
total_amount = float(input("Enter the total amount: "))
total_people = int(input("Enter the total number of people: "))
amount_due = split_check(total_amount, total_people)
except ValueError as err:
print("Please enter a valid number")
print("({})".format(err))
else:
print("Total amount to be paid by each person is: {}$ ".format(amount_due))
maybe this will be a way to solve your probelm

Suresh Nagalla
1,648 Points1/ You have defined 2 except statments for handling ValueError . 2/ Whenever ValueError exception raised , it will get caught by the 1st except statement , then program will get terminated ..Due to which you are not seeing "Please enter a valid number" in your output.
axelh
10,077 Pointsaxelh
10,077 PointsHi there!
I fiddled around with it a bit, see below! If there's anything, be sure to give a shout :)
You can use the ``` before and after your code to mark it as code. You can check the markup cheatsheet if you click on the 'Markdown Cheatsheet' when typing your comment or message beneath the typing area itself.