Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial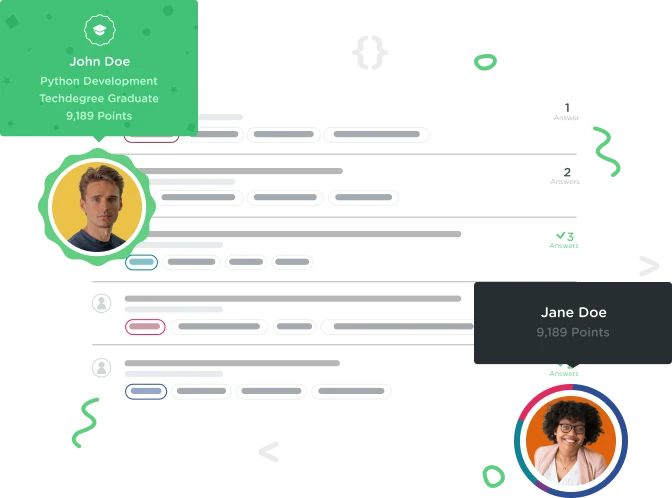

Patrick Castle
Courses Plus Student 14,591 PointsHandling Routes in Node.js
When handling routes I notice that the Node.js server will call every route function for each request. I'm wondering if it would be better to use if statements or a switch statement in the main server loop so that only the required function is called for each request.
var router = require("./router.js");
var http = require('http');
http.createServer(function (request, response) {
router.home(request, response);
router.user(request, response);
}).listen(8080);
console.log('Server running at http://<workspace-url>/');
If you notice in the above, it looks like each request would call router.home() and then router.user(). My immediate reaction is that this looks inefficient as you'll only actually want one of those called.
Admittedly each of those functions immediately starts with an if statement to either reject or further process the request so it's almost the same thing as putting if statements in that main createServer() function. However, wouldn't the unnecessary function calls themselves be a waste? Or are there more problems with putting additional logic inside the main request handler?
1 Answer

Kostas Oreopoulos
15,184 PointsNo. its better to do it this way, handle the logic inside the router, because its simpler to write, reorder, test and reuse. Actually this is how express.js framework works using the notion of middleware (aka actions that need to be performed in a certain order.
The logic is, you take a request and you want to pass it over certain actions. Its better to keep let the actions decide if they should be applied or not. That way you can take that action and put it in a later project as is and reuse it with zero effort.