Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial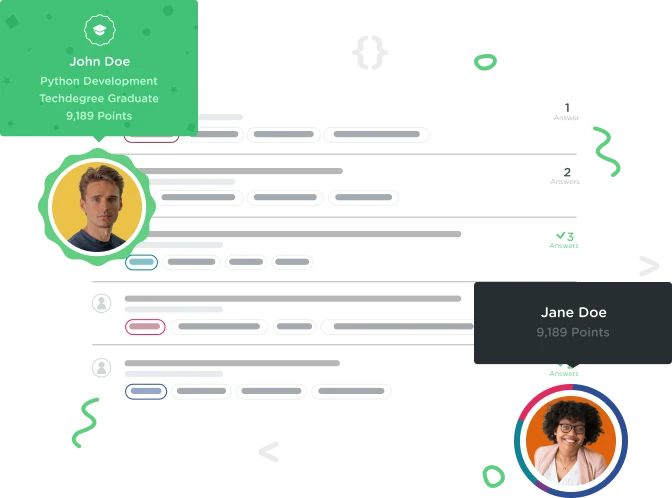
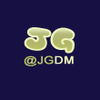
Jonathan Grieve
Treehouse Moderator 91,253 PointsHangman game always reports a hit
Hi all,
So I've got a slight problem here. I'm almost done, there's no console errors but when I test the game in workspaces, the compiler always reports a hit, no matter what letter I try.
From what I can see the code is correct, the prompt messages appears correctly and the guesses method is called on the Prompter object. Something tiny is causing a big problem here :) Here's all my code so far.
hangman.java
public class Hangman {
public static void main(String[] args) {
// Enter amazing code here:
//Supply a string to start the game.
Game game = new Game("treehouse");
//The following code calls the prompterForGuess method with a new Prompter object.
Prompter prompter = new Prompter(game);
boolean isHit = prompter.promptForGuess();
if(isHit) {
System.out.println("We got a Hit!");
} else {
System.out.println("Whoops that was a miss");
}
}
}
game.java
public class Game {
//variable declared but not initialised
private String mAnswer;
private String mHits;
private String mMisses;
//constructor function taking answer in form of a string
public Game(String answer) {
mAnswer = answer;
mHits = "";
mMisses = "";
}
public boolean applyGuess(char letter) {
boolean isHit = mAnswer.indexOf(letter) >= 0;
if (isHit) {
mHits += letter;
} else {
mMisses += letter;
}
return true;
}
}
prompter.java
//Import package
import java.io.Console;
public class Prompter {
//private member variable
private Game mGame;
//constructor function
public Prompter (Game game) {
//set member variable equal to what is passed in
mGame = game;
}
//a prompter method for the user to guess new letters
public boolean promptForGuess() {
Console console = System.console();
String guessAsString = console.readLine("Enter a letter: ");
//define new guess in a char variable.
char guess = guessAsString.charAt(0);
return mGame.applyGuess(guess);
}
}
1 Answer

Seth Kroger
56,414 PointsIn your applyGuess() method:
public boolean applyGuess(char letter) {
boolean isHit = mAnswer.indexOf(letter) >= 0;
if (isHit) {
mHits += letter;
} else {
mMisses += letter;
}
return true;
}
You are always returning true at the end. I think you put return true;
in as a stub so your IDE/compiler wouldn't complain about it before you wrote the rest but forgot to return isHit when you finished.
Jonathan Grieve
Treehouse Moderator 91,253 PointsJonathan Grieve
Treehouse Moderator 91,253 PointsPretty obvious when you think about it. Guess I just needed a break from the screen, but yes I managed to solve it by returning true and then false in the else block. :)