Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial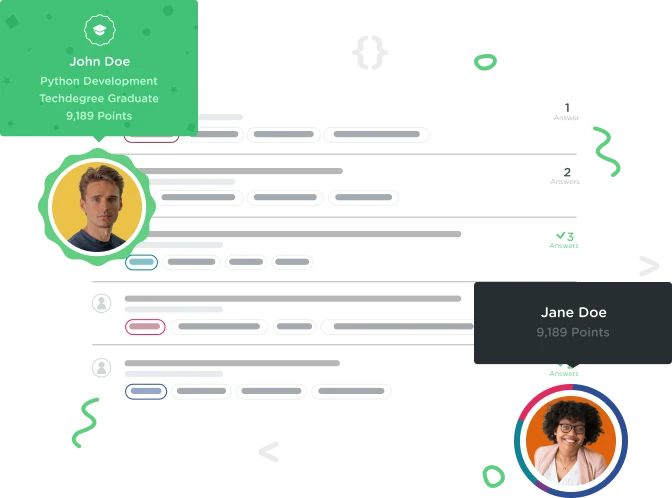

Sahil Gangele
6,851 PointsHangman Question
import java.io.Console;
public class Prompter {
private Game mGame;
public Prompter(Game game) {
mGame = game;
}
public boolean promptForGuess() {
Console console = System.console();
String guessAsString = console.readLine("Enter a letter: ");
char guess = guessAsString.charAt(0);
return mGame.applyGuess(guess);
}
}
In the Prompter.java file, I don't understand what is going on when the constructor is made. Why is the constructor made, and what is its purpose? Also, I don't understand the return method and how it works, when 'mGame' is used. Why do you need to use 'mGame"?
It would be much appreciated if a line by line breakdown could be done. I just need to grasp the understanding of what each line is doing.
Thanks!
1 Answer
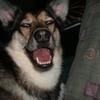
Isaiah Marin
11,971 PointsHi Sahil,
Constructors are normally called when you create an object (note: there is a default constructor when you do not code one yourself). Also note, constructors will not return anything but it is used to assign member variables.
For example: Say we have a Game object and we want to pass it to a Prompter object (a reason to do this is to allow a object to have access to the other object's class methods).
Game game = new Game(); // here we create a game object
Prompter prompt = new Prompter(game); // here we pass the game object as an argument in the Prompter constructor
Notice when we create the Prompter object called prompt and we pass the Game object named game through the Prompter constructor. Since we passed the object game through the constructor, it will then use the constructor you coded. This will assign the prompt's object mGame to game (thus you now have access to game through your mGame object). Which is what you see in the code:
public Prompter(Game game) {
mGame = game;
}
Some of the reasons for doing this is to allow a class object to gain access to another class object. An example to this situation is the line of code
return mGame.applyGuess(guess);
This will return mGame's method applyGuess() while passing the argument guess. But since promptForGuess() will have to return a Boolean value we know it will return a Boolean. But it will do so from the result in returning whatever the method applyGuess(guess) will return.
Hope this helps,
Isaiah Marin
Cameron Raw
15,473 PointsCameron Raw
15,473 PointsThis is such a good explanation and yet I still feel a bit lost. So, on the Prompter.java file, we have a constructor. We're passing the constructor 'game' which is the same game we construct from the Hangman.java file? So mGame is the Prompter class' member variable representing the actual game?
Am I right in thinking that...
is referencing the same object as
Game game = new Game("treehouse");
Sorry to hijack this question but when Craig quickly types that bit up in Prompter.java and says it's to 'pull out the game logic' I just had no clue what was going on.