Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial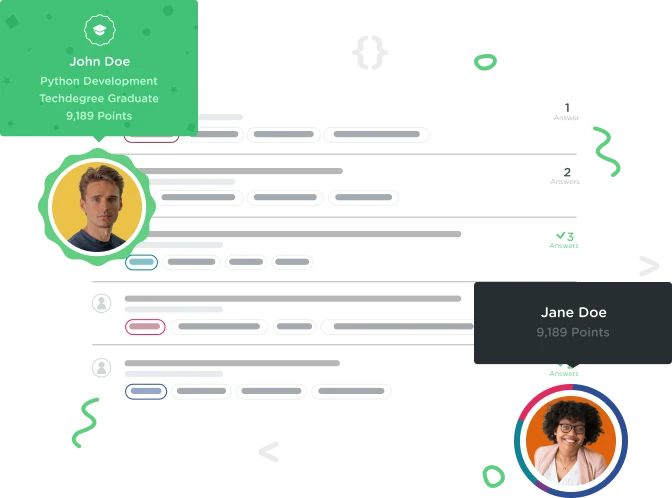

Angelus Miculek
6,147 Pointshappy birthday rubik
These challenges were excellent practice!
Here's my solutions (my answer to challenge 3 is probably inefficient, but it's simple enough).
from dateutil import relativedelta
import datetime
from utils import no_s_if_1
def date_parser(person):
format_string = "%Y-%m-%d"
birthday_dt = datetime.datetime.strptime(person['birthday'], format_string)
return birthday_dt
def diff_func(person):
birthday_dt = date_parser(person)
now = datetime.datetime.now()
birthday_this_year = birthday_dt.replace(year=now.year)
difference = birthday_this_year - now
return difference
def upcoming_birthdays(people_list, days):
# TODO: write code that finds all upcoming birthdays in the next 90 days
# 90 is passed in as a parameter from menus.py
# Template:
# PERSON turns AGE in X days on MONTH DAY
# PERSON turns AGE in X days on MONTH DAY
# print("Upcoming Birthdays function")
# print(people_list)
# pass
sorted_bd_list = []
sorted_person_list = []
for person in people_list:
difference = diff_func(person)
sorted_bd_list.append(difference.days)
sorted_bd_list.sort()
for dif in sorted_bd_list:
for person in people_list:
difference = diff_func(person)
if difference.days == dif:
sorted_person_list.append(person)
for person in sorted_person_list:
birthday_dt = date_parser(person)
now = datetime.datetime.now()
birthday_this_year = birthday_dt.replace(year=now.year)
difference = birthday_this_year - now
turning_age = relativedelta.relativedelta(now, birthday_dt).years + 1
if 0 <= difference.days <= days:
print(f"{person['name']} turns {turning_age} in {no_s_if_1(difference.days, 'days')} on {birthday_this_year.strftime('%B %d')}")
if -365 <= difference.days <= -275:
hey = difference.days + 365
print(f"{person['name']} turns {turning_age} in {no_s_if_1(hey, 'days')} on {birthday_this_year.strftime('%B %d')}")
print(sorted_bd_list)
# print(sorted_person_list)
# print(people_list)
def display_age(person):
# TODO: write code to display the age of person
# Template:
# PERSON is X years, X months, and X days old
birthday_dt = date_parser(person)
today = datetime.date.today()
difference = relativedelta.relativedelta(today, birthday_dt)
print(f"{person['name']} is {no_s_if_1(difference.years, 'years')}, {no_s_if_1(difference.months, 'months')}, and {no_s_if_1(difference.days, 'days')} old")
# print(person)
# pass
def display_age_difference(people):
# TODO: write the code to display the age difference between people
# Template:
# PERSON is older
p0_dt = date_parser(people[0])
p1_dt = date_parser(people[1])
if p0_dt < p1_dt:
difference = relativedelta.relativedelta(p1_dt, p0_dt)
print(f"{people[0]['name']} is older")
else:
difference = relativedelta.relativedelta(p0_dt, p1_dt)
print(f"{people[1]['name']} is older")
# print(difference)
print(f"{people[0]['name']} and {people[1]['name']}'s age difference is: {no_s_if_1(difference.years, 'years')}, {no_s_if_1(difference.months, 'months')}, and {no_s_if_1(difference.days, 'days')}")
# print(people)
# pass
Have a great day!
1 Answer
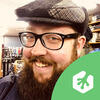
Rohald van Merode
Treehouse StaffGlad to hear you've enjoyed this additional practice 😃
Thanks for sharing your solution!