Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial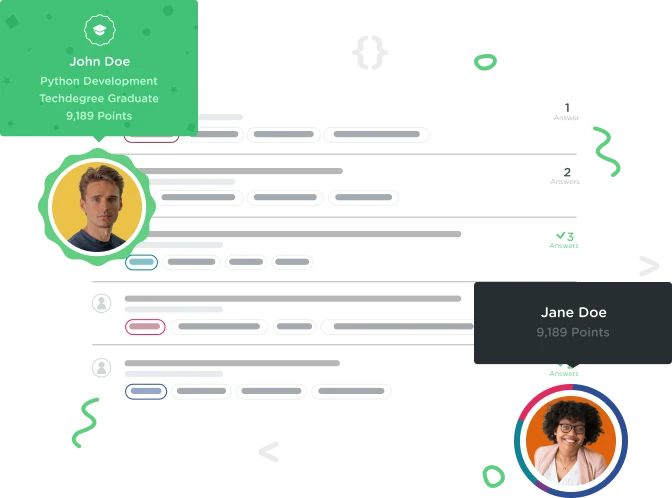

Dave Laffan
4,604 PointsHarder Time Machine
Hi. I've already passed this challenge with
def time_machine(int1, str1):
if str1 == 'minutes':
return starter + datetime.timedelta(minutes=int1)
elif str1 == 'hours':
return starter + datetime.timedelta(hours=int1)
elif str1 == 'days':
return starter + datetime.timedelta(days=int1)
elif str1 == 'years':
return starter + datetime.timedelta(days=int1*365)
I've tried simplifying it with
def time_machine(int1, str1):
return starter + datetime.timedelta(str1=int1)
and that doesn't work. I kind of get why, I think it's because I'm trying to pass a string when it's expecting days/weeks/months - but not sure how to get around this?
I'll know I'll need a quick if/elif for the year, but wanted to simplify the minutes/hours/days somehow?
1 Answer

Wade Williams
24,476 PointsI like your style, passing something then making it better. What you're looking for is keyword unpacking which you can do with a dictionary.
def time_machine(int1, str1):
time_dict = {str1: int1}
if str1 == "years":
time_dict = {"days": int1*365}
return starter + datetime.timedelta(**time_dict)
You can also just pass in a dictionary directly into the function as well, which would be really clean if we didn't have to worry about turning "years" into "days".
def time_machine(int1, str1):
if str1 == "years":
str1 = "days"
int1 *= 365
return starter + datetime.timedelta(**{str1: int1})
Here's a good article on * and ** in function parameters:
http://agiliq.com/blog/2012/06/understanding-args-and-kwargs/
Dave Laffan
4,604 PointsDave Laffan
4,604 PointsHi Wade, really helpful, thank you :)
Erik Burmeister
Python Web Development Techdegree Graduate 17,108 PointsErik Burmeister
Python Web Development Techdegree Graduate 17,108 PointsWade Williams, thanks for taking the time to answer. I was in the same boat as Dave. Your explanation really cleared things up.