Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial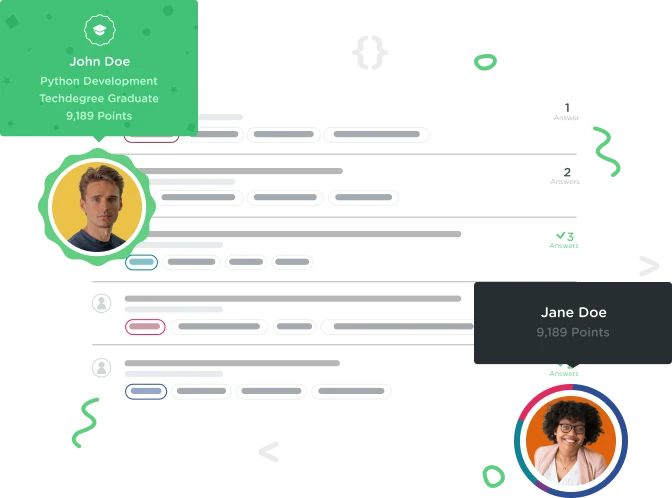

Adnan Fateh
4,396 PointsHarder Time Machine - Bummer: local variable 'td' referenced before assignment
My code yields the following error: Bummer: local variable 'td' referenced before assignment
When I flip the arguments in the "time_machine" function to "(num, time_string)", the code passes the challenge. I don't understand why. I think it might work the first way if I assigned the td variable as some initial value- but I get all kinds of errors for trying that. Can someone help me understand why it works one way and not the other.
import datetime
starter = datetime.datetime(2015, 10, 21, 16, 29)
# Remember, you can't set "years" on a timedelta!
# Consider a year to be 365 days.
## Example
# time_machine(5, "minutes") => datetime(2015, 10, 21, 16, 34)
def time_machine(time_string, num):
if time_string == 'minutes':
td = datetime.timedelta(minutes=num)
elif time_string == 'hours':
td = datetime.timedelta(hours=num)
elif time_string == 'days':
td = datetime.timedelta(days=num)
elif time_string == 'years':
td = datetime.timedelta(days=num*365)
return starter + td
2 Answers
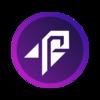
Rocket Dollar Invest
7,129 PointsA simple solution for this problem is to first check if the string is 'years' and if so change the string to 'days' since timedelta will not except years as an argument. Then multiply the integer by 365 to get the equivalent number of days for the given number of years. Finally, return the duration as the difference of the starter datetime and the timedelta of the string and integer passed to the method as a literal dictionary with the ** prefix operator to unpack the dictionary.
import datetime
starter = datetime.datetime(2015, 10, 21, 16, 29)
def time_machine(integer, string):
if string == 'years':
string = 'days'
integer *= 365
return starter + datetime.timedelta(**{string: integer})
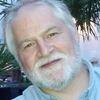
Jeff Muday
Treehouse Moderator 28,722 Points- Change your last elif to a simple else clause, then td will be instantiated.
- Reverse the order of time_string and num
def time_machine(num, time_string):
if time_string == 'minutes':
td = datetime.timedelta(minutes=num)
elif time_string == 'hours':
td = datetime.timedelta(hours=num)
elif time_string == 'days':
td = datetime.timedelta(days=num)
else:
td = datetime.timedelta(days=num*365)
return starter + td
Jeff Muday
Treehouse Moderator 28,722 PointsJeff Muday
Treehouse Moderator 28,722 PointsI like how you think about this. You already have the instincts that will serve you well as a developer!
I definitely agree that capturing days/years together would work as a good default conversion unit. Though the Challenge unit test may include "hours" and "minutes" specifications, so it is good practice to include those cases.